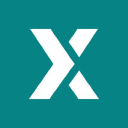
Poloniex
CryptocurrencyAn Overview of the Poloniex Public API
The Poloniex API allows you to access a wide variety of data related to trading on the Poloniex exchange, including ticker data, order book information, trade history, and more. In this article, we'll take a look at some sample JavaScript code that demonstrates how to use the Poloniex API to retrieve data and perform various functions.
Getting Started
Before we dive into the sample code, let's first take a look at how to set up the API and obtain an API key and secret. To get started, simply follow these steps:
- Sign up for an account on Poloniex.
- Log in to your account and navigate to the API Keys page.
- Generate a new API key and secret.
- Be sure to keep your secret key safe and secure.
Once you have your API key and secret, you can begin using the API to retrieve data from the exchange.
Sample Code
To get started, we'll first need to use a JavaScript library to send HTTP requests to the Poloniex API. In this example, we'll be using the request
library, which can be installed using npm. To install the request
library, simply run the following command:
npm install request
Once you've installed the request
library, you can begin using it to send requests to the Poloniex API.
Example 1: Retrieving Ticker Data
To retrieve ticker data for a particular cryptocurrency on the Poloniex exchange, you can use the following code:
const request = require('request');
const currencyPair = 'BTC_ETH';
const options = {
url: `https://poloniex.com/public?command=returnTicker¤cyPair=${currencyPair}`,
json: true,
};
request(options, (error, response, body) => {
if (!error && response.statusCode === 200) {
console.log(body);
}
});
In this example, we're retrieving ticker data for the BTC_ETH
currency pair. We're using the returnTicker
API command to retrieve this data, and we're using the json
option to automatically parse the response as JSON.
Example 2: Retrieving Order Book Data
To retrieve order book data for a particular currency pair, you can use the following code:
const request = require('request');
const currencyPair = 'BTC_ETH';
const depth = 10;
const options = {
url: `https://poloniex.com/public?command=returnOrderBook¤cyPair=${currencyPair}&depth=${depth}`,
json: true,
};
request(options, (error, response, body) => {
if (!error && response.statusCode === 200) {
console.log(body);
}
});
In this example, we're retrieving order book data for the BTC_ETH
currency pair. We're using the returnOrderBook
API command to retrieve this data, and we're specifying a depth
of 10 levels.
Example 3: Retrieving Trade History
To retrieve trade history for a particular currency pair, you can use the following code:
const request = require('request');
const currencyPair = 'BTC_ETH';
const start = 1609459200;
const end = 1612137600;
const options = {
url: `https://poloniex.com/public?command=returnTradeHistory¤cyPair=${currencyPair}&start=${start}&end=${end}`,
json: true,
};
request(options, (error, response, body) => {
if (!error && response.statusCode === 200) {
console.log(body);
}
});
In this example, we're retrieving trade history data for the BTC_ETH
currency pair. We're using the returnTradeHistory
API command to retrieve this data, and we're specifying a start
and end
timestamp for the trade data.
Conclusion
In this article, we've looked at some sample JavaScript code that demonstrates how to use the Poloniex API to retrieve data and perform various functions. Whether you're interested in retrieving ticker data, order book information, or trade history, the Poloniex API provides a wide variety of functionality for traders and developers alike.