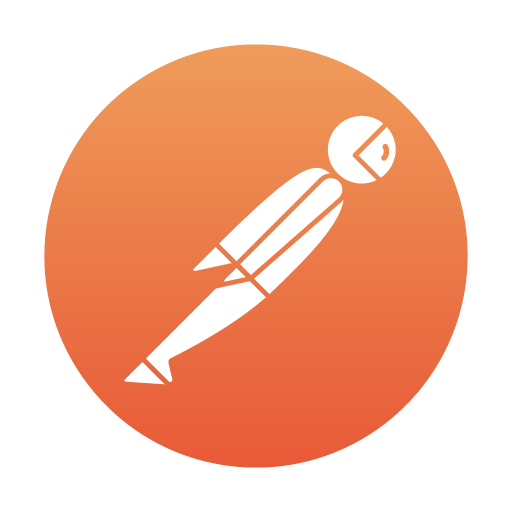
Postman
DevelopmentIntroduction
APIs or Application Programming Interfaces play a critical role in modern-day software development. They allow different applications to communicate with each other and share data effectively. In this blog post, we will take a closer look at the public API provided by Postman and examine some examples of how to interact with it using JavaScript.
Postman API
The Postman API allows developers to access and manage their Postman resources programmatically. With this API, you can create and update collections, environments, mock servers, and more. It allows you to interact with your Postman data to automate workflow and integrate Postman with other tools in your development environment.
Prerequisites
Before we dive into JavaScript code examples, make sure you have the following:
- A Postman account
- A Postman API key
- Knowledge of the basics of JavaScript
Example Code
Here are some examples of how to interact with the Postman API using JavaScript:
Getting all collections
fetch('https://api.getpostman.com/collections', {
headers: {
'X-Api-Key': '<your Postman API key>'
}
}).then(res => res.json())
.then(data => console.log(data.collections));
Creating a new collection
const newCollection = {
'collection': {
'info': {
'name': 'New Collection'
}
}
};
fetch('https://api.getpostman.com/collections', {
method: 'POST',
headers: {
'X-Api-Key': '<your Postman API key>',
'Content-Type': 'application/json'
},
body: JSON.stringify(newCollection)
}).then(res => res.json())
.then(data => console.log(data));
Updating an existing collection
const updatedCollection = {
'collection': {
'info': {
'name': 'Updated Collection'
}
}
};
fetch('https://api.getpostman.com/collections/<collection_id>', {
method: 'PUT',
headers: {
'X-Api-Key': '<your Postman API key>',
'Content-Type': 'application/json',
},
body: JSON.stringify(updatedCollection)
}).then(res => res.json())
.then(data => console.log(data));
Deleting a collection
fetch('https://api.getpostman.com/collections/<collection_id>', {
method: 'DELETE',
headers: {
'X-Api-Key': '<your Postman API key>'
},
}).then(res => console.log(res.status));
Conclusion
Accessing and managing your Postman resources programmatically can help automate your workflow and enhance your development experience. In this blog post, we examined the Postman API and explored some code examples for interacting with it using JavaScript. With these examples, you should be able to get started with integrating Postman into your own development environment.