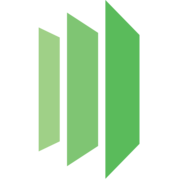
PrexView
Documents & ProductivityIntroduction to PreXView API
PreXView is a powerful document automation solution that enables enterprises to easily generate and manage documents using a simple web interface. Their API provides developers with access to powerful document generation functionalities that can be integrated into your web or mobile app. PreXView API is available in several programming languages, including JavaScript. In this article, we will go over some of the most important API examples in JavaScript.
Prerequisites
Before we get started, you will need to have an account on PreXView and API Key. You can create a free account here. Once you have created your account, you can generate your API Key from the dashboard.
Install
To use PreXView API in your JavaScript project, first, you will need to install the prexview package using npm.
npm install prexview --save
Examples
1. Generating a PDF with PreXView API
The following code generates a PDF document using PreXView API.
const PrexView = require('prexview');
const fs = require('fs');
const prexview = new PrexView({
apiKey: '<YOUR_API_KEY>', // replace with your own API key
});
const template = `<template>
<pdf>
<h3>Hello World</h3>
<p>This is a sample PDF generated using PreXView API</p>
</pdf>
</template>`;
prexview.generateDocument(template, 'pdf')
.then((response) => {
const fileName = 'hello-world.pdf';
fs.writeFile(fileName, response.document, (err) => {
if (err) throw err;
console.log(`${fileName} has been saved!`);
});
})
.catch((err) => console.error(err));
2. Uploading a Template to PreXView API
The following code uploads a template to PreXView API.
const PrexView = require('prexview');
const prexview = new PrexView({
apiKey: '<YOUR_API_KEY>', // replace with your own API key
});
const template = `<template>
<pdf>
<h3>Hello World</h3>
<p>This is a sample PDF generated using PreXView API</p>
</pdf>
</template>`;
const fileName = 'hello-world-template.xml';
prexview.uploadTemplate(template, fileName)
.then((response) => console.log(response))
.catch((err) => console.error(err));
3. Generating a PDF from a Template with Variables
The following code generates a PDF document using PreXView API from a template with variables.
const PrexView = require('prexview');
const fs = require('fs');
const prexview = new PrexView({
apiKey: '<YOUR_API_KEY>', // replace with your own API key
});
const variables = {
name: 'PreXView',
};
prexview.generateDocumentFromTemplate('template.xml', 'pdf', variables)
.then((response) => {
const fileName = 'hello-prexview.pdf';
fs.writeFile(fileName, response.document, (err) => {
if (err) throw err;
console.log(`${fileName} has been saved!`);
});
})
.catch((err) => console.error(err));
4. Generating a Document from a Remote URL
The following code generates a PDF document using PreXView API from a remote URL.
const PrexView = require('prexview');
const fs = require('fs');
const prexview = new PrexView({
apiKey: '<YOUR_API_KEY>', // replace with your own API key
});
const remoteUrl = 'https://prexview.com/docs/hello-world-template.xml';
const variables = {
name: 'PreXView',
};
prexview.generateDocumentFromRemoteUrl(remoteUrl, 'pdf', variables)
.then((response) => {
const fileName = 'hello-prexview.pdf';
fs.writeFile(fileName, response.document, (err) => {
if (err) throw err;
console.log(`${fileName} has been saved!`);
});
})
.catch((err) => console.error(err));
Conclusion
PreXView API provides developers with a simple and powerful way to generate and manage documents in their web or mobile app. In this article, we have gone through some of the most important examples in JavaScript. The possibilities are endless with PreXView API, and we encourage you to explore further and integrate it into your next project.