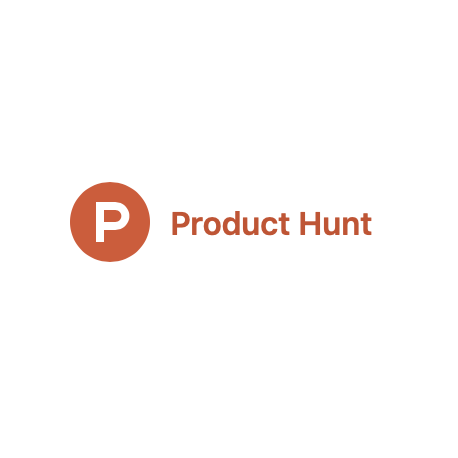
Product Hunt API
DevelopmentProduct Hunt now (finally) has its own api. Through this api you can access posts of the day, tech posts, filtered with a category, add a post etc. You can fetch list of users. Fetch comments, threads, notifications and even upvote your favorite products. The api lets you provide complete product hunt experience at one place. Use the api to get a taste !
📚 Documentation & Examples
Everything you need to integrate with Product Hunt API
🚀 Quick Start Examples
// Product Hunt API API Example
const response = await fetch('https://api.producthunt.com/v1/docs', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using Product Hunt API with JavaScript
If you’re looking to access Product Hunt’s data programmatically, you’ve come to the right place. Product Hunt’s API uses RESTful endpoints and returns JSON responses. In this blog post, we’ll explore how to use the Product Hunt API with JavaScript.
Authentication
Before you can start making requests to the Product Hunt API, you need to authenticate yourself by obtaining an access token. You can read the documentation on how to obtain an access token here.
Getting Started
The Product Hunt API has several endpoints. You can read the full documentation on the available endpoints here.
To get started, let’s make a request to the /v1/posts
endpoint, which returns the current day’s top posts. We’ll use the fetch
function to make the request:
fetch('https://api.producthunt.com/v1/posts', {
headers: {
'Accept': 'application/json',
'Authorization': 'Bearer {ACCESS_TOKEN}',
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));
Replace {ACCESS_TOKEN}
with your own access token. This code will log the JSON response from the API to the console.
Example: Search for Products
Let’s say we want to search for products related to “design”. We can use the /v1/api/search
endpoint to make the request:
fetch('https://api.producthunt.com/v1/api/search?query=design&type=product', {
headers: {
'Accept': 'application/json',
'Authorization': 'Bearer {ACCESS_TOKEN}',
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));
This will log an array of products related to “design” to the console.
Example: Get Product Details
Let’s say we want to get details about a specific product. We can use the /v1/products/:id
endpoint to make the request:
fetch('https://api.producthunt.com/v1/products/123', {
headers: {
'Accept': 'application/json',
'Authorization': 'Bearer {ACCESS_TOKEN}',
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));
Replace 123
with the ID of the product you want to get details for. This will log the details of the specified product to the console.
Conclusion
In this blog post, we explored how to use the Product Hunt API with JavaScript. We covered authentication, making requests to the API, and provided some examples. Now that you’re familiar with the basics, you can start building your own applications that use Product Hunt’s data.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes