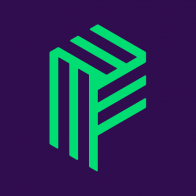
Pusher Beams
DevelopmentUsing Pusher Beams API in JavaScript
Pusher Beams is a powerful push notification API that allows developers to send push notifications to mobile devices in real-time. In this blog post, we will explore how to use Pusher Beams in JavaScript by looking at some example code snippets.
Getting started
Before we can start using Pusher Beams in JavaScript, we first need to obtain our credentials from the Pusher dashboard. Once we have our credentials, we can use the Pusher Beams SDK to send push notifications.
Example code
const PushNotifications = require('@pusher/push-notifications-web');
const beamsClient = new PushNotifications({
instanceId: 'YOUR_INSTANCE_ID',
key: 'YOUR_KEY',
});
beamsClient.publishToInterests(['hello'], {
web: {
notification: {
title: 'Hello!',
body: 'World!',
},
},
});
In the code above, we first import the Pusher Beams SDK and initialize it with our credentials. We then use the publishToInterests
method to send a notification to all subscribers who are interested in the topic "hello". We define the content of the notification in the web
object, which contains the notification
object that specifies the title
and body
of the notification.
beamsClient.addDeviceInterest('hello');
beamsClient.getDeviceInterests().then(interests => {
console.log(interests);
});
The above code adds the interest "hello" to the current device and retrieves all the interests of the device. This can be useful if we want to target notifications to specific devices.
beamsClient.setUserId('USER_ID');
beamsClient.publishToUsers(['USER_ID'], {
web: {
notification: {
title: 'Hello!',
body: 'World!',
},
},
});
In this code snippet, we set the "USER_ID" of the current user and send a notification to that user. The publishToUsers
method sends the notification to all devices that are associated with the specified user ID. We can also pass additional data in the notification payload.
Conclusion
Pusher Beams is an excellent API that simplifies the process of sending push notifications to mobile devices in real-time. In this blog post, we explored how to use Pusher Beams in JavaScript by looking at some example code snippets. We hope that this post has been helpful in demonstrating the power and flexibility of Pusher Beams in JavaScript applications.