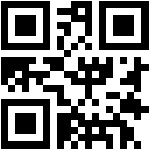
π Documentation & Examples
Everything you need to integrate with QR code API
π Quick Start Examples
// QR code API API Example
const response = await fetch('http://goqr.me/api/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using the GOQR Public API in JavaScript
GOQR provides a public API for generating QR codes. This API allows developers to generate QR codes directly from their applications. In this tutorial, we will explore how to use the GOQR public API in JavaScript.
API Endpoints
The GOQR Public API has the following endpoint:
Base URL: http://api.qrserver.com/v1/create-qr-code/
Generating QR Codes
To generate a QR code, you will need to pass in the URL of the website or the desired data to be encoded in the QR code. Here is an example code snippet to generate a QR code from the data:
const qrCodeData = 'https://www.example.com';
const qrCodeURL = `http://api.qrserver.com/v1/create-qr-code/?data=${qrCodeData}&size=100x100`;
Here, we are using string interpolation to dynamically create the URL to generate the QR code. We have provided the size of the QR code as 100x100 pixels, but this can be adjusted to any value as needed.
Displaying the QR Code
Once the URL of the QR code has been generated, it can be used to display the QR code on a webpage. Here is an example code snippet to display the QR code on a webpage:
const imgElement = document.createElement('img');
imgElement.src = qrCodeURL;
document.body.appendChild(imgElement);
Here, we are creating an img
element and setting its src
property to the generated QR code URL. We are then appending the img
element to the body of the HTML document. This creates an image on the webpage that displays the QR code.
Conclusion
The GOQR Public API is a powerful tool for generating QR codes directly from your application. With the help of this tutorial, you can easily generate QR codes using JavaScript. The possibilities are endless with the GOQR Public API, and you can use it to generate QR codes for various applications.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes