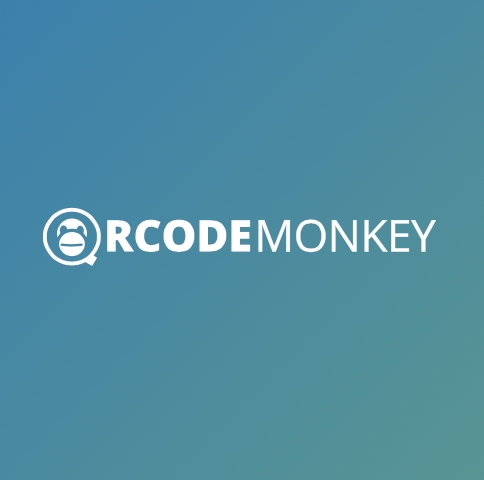
Qrcode monkey API
DevelopmentHow to Use QR Code Monkey API with Logo
QR Code Monkey is a website that offers a free, fast, and easy-to-use QR code generator. With its API, users can easily generate QR codes with logo programmatically. In this blog post, we'll be looking at how to use the QR Code Monkey API with logo using JavaScript.
Generating a QR Code with Logo
To generate a QR code with a logo, you'll need to sign up for a free API key on QR Code Monkey's website. Once you have your API key, you can start generating QR codes with logos by sending a GET request to:
https://www.qrcode-monkey.com/api/qr/custom?logo_url=<logo_url>&qr_code_text=<qr_code_text>&logo_size=<logo_size>&qr_code_size=<qr_code_size>&api_key=<api_key>
Here's a breakdown of the different parameters:
logo_url
: The URL of the image you want to use as the logo.qr_code_text
: The text you want to encode as a QR code.logo_size
: The size of the logo in pixels (default is 100x100).qr_code_size
: The size of the QR code in pixels (default is 500x500).api_key
: Your API key.
Here's an example of how to use the QR Code Monkey API with logo in JavaScript:
const logoUrl = 'https://www.qrcode-monkey.com/img/qrcode-monkey.png';
const qrCodeText = 'Hello, World!';
const logoSize = 150;
const qrCodeSize = 600;
const apiKey = 'your-api-key';
const qrCodeGeneratorUrl = `https://www.qrcode-monkey.com/api/qr/custom?logo_url=${logoUrl}&qr_code_text=${qrCodeText}&logo_size=${logoSize}&qr_code_size=${qrCodeSize}&api_key=${apiKey}`;
fetch(qrCodeGeneratorUrl)
.then(response => response.json())
.then(data => {
const qrCodeImg = document.createElement('img');
qrCodeImg.src = data.image_url;
document.body.appendChild(qrCodeImg);
});
In this example, we first define the URL of the logo, the text to encode, the size of the logo, the size of the QR code, and our API key. We then generate the URL to the QR code generator API by interpolating these values into the template string. Finally, we make a GET request to the generated URL using the fetch
API, parse the JSON response, and insert the resulting QR code into the document body as an image.
Conclusion
In this blog post, we've looked at how to use the QR Code Monkey API with logo using JavaScript. With its simple API, QR Code Monkey makes it easy for developers to generate QR codes with logos programmatically. Whether you're building an app that needs to generate QR codes on-the-fly or simply need to generate QR codes for print, QR Code Monkey is a great tool to have in your arsenal.