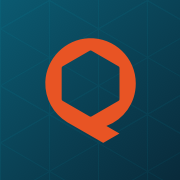
Quandl API
Data AccessGet millions of financial and economic datasets from hundreds of publishers via a single free API.
π Documentation & Examples
Everything you need to integrate with Quandl API
π Quick Start Examples
// Quandl API API Example
const response = await fetch('https://www.quandl.com/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using Quandl API to Retrieve Financial Data
Quandl is a platform for financial, economic, and alternative data that provides public APIs for developers to access data from a variety of sources. In this blog post, we'll take a look at how we can use the Quandl API to retrieve financial data using JavaScript.
Getting Started with Quandl API
To get started with the Quandl API, you'll need to create a free account and get an API key. You can do this by visiting the Quandl website and signing up for an account.
Once you have an API key, you can use it to access the Quandl API. The API provides several endpoints that allow you to retrieve data from different datasets. You can find the complete documentation for the Quandl API on the Quandl website.
Retrieving Data from Quandl API
You can retrieve financial data from the Quandl API by sending a request to the relevant API endpoint. To do this using JavaScript, you can use the fetch
function to send an HTTP request to the API endpoint and retrieve the data.
Here's an example code snippet that shows how you can retrieve stock prices for Apple Inc. using the Quandl API:
const apiKey = 'YOUR_API_KEY';
const stockSymbol = 'AAPL';
const apiUrl = `https://www.quandl.com/api/v3/datasets/WIKI/${stockSymbol}.json?api_key=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
// Do something with the retrieved data
})
.catch(error => {
console.log('Error:', error);
});
In this code snippet, you need to replace the YOUR_API_KEY
placeholder with your actual API key. You also need to specify the stock symbol for the company whose data you want to retrieve.
The code sends an HTTP request to the Quandl API endpoint and retrieves the stock data for the specified stock symbol. The retrieved data is in JSON format, and you can use the data
variable in the response callback function to access the data.
Conclusion
Using the Quandl API, you can retrieve financial data for different companies and industries. In this blog post, we've seen how you can use the Quandl API to retrieve stock prices for a given company using JavaScript. You can refer to the Quandl API documentation for more information on available endpoints and datasets.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes