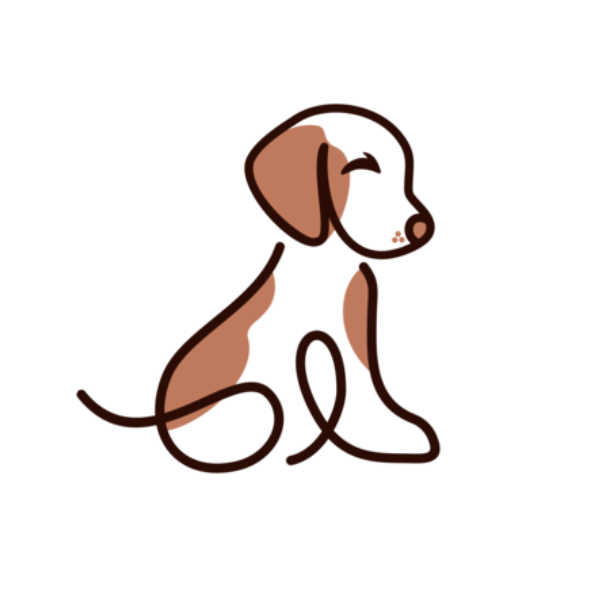
RandomDog
AnimalsFetch random image of dogs. These are high resolution wallpapers. You can build your wallpapers website or make a kids game around this api. Or if you are just starting to code, put dog faces on the front of your card laid out in a grid format. Try to play with it to sharpen your web building skills. It is free and unlimited.
📚 Documentation & Examples
Everything you need to integrate with RandomDog
🚀 Quick Start Examples
// RandomDog API Example
const response = await fetch('https://random.dog/woof.json?ref=apilist.fun', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using Random.dog API in JavaScript
Random.dog is a public API that provides random dog images in various formats. In this blog post, we will learn how to use the Random.dog API in JavaScript.
Getting started
Before we begin, make sure you have access to an up-to-date web browser with JavaScript enabled. You will also need to have knowledge of JavaScript and how to use standard web development tools.
API Endpoints
The Random.dog API provides only one endpoint, which returns a random dog image. The endpoint is as follows:
https://random.dog/woof.json
This API endpoint returns a JSON object that contains a URL to a randomly selected dog image. The JSON response will look like this:
{
"url": "https://random.dog/3a2d972a-ef3e-4c43-9394-397b3f0f98e4.jpg"
}
Usage
There are a few steps required to use the Random.dog API in your JavaScript application:
-
Build the API request URL: To get a random dog image, we need to send a GET request to the Random.dog API endpoint. We can do that using JavaScript's
fetch
method. The URL for the request is simplyhttps://random.dog/woof.json
. -
Send the API request: Once we have built the API request URL, we can send the request using the
fetch
method. -
Parse the API response: After sending the API request, we need to parse the response to extract the URL for the dog image.
-
Display the dog image: Finally, we can use the URL from the API response to display the dog image in our application.
Here is an example JavaScript code that demonstrates how to use the Random.dog API:
fetch("https://random.dog/woof.json")
.then(response => response.json())
.then(data => {
const dogImage = document.createElement("img");
dogImage.src = data.url;
document.body.appendChild(dogImage);
})
.catch(error => console.error(error));
In this example, we use the fetch
method to send a GET request to the Random.dog API endpoint. Once we get the response, we extract the URL for the dog image by parsing the JSON response. Then we create an img
element in the DOM, set its src
attribute to the URL, and append it to the body
of the page.
Conclusion
In conclusion, using the Random.dog API in JavaScript is a simple and straightforward process. With just a few lines of code, you can retrieve random dog images and display them in your application. If you want to learn more about the API, you can visit the official website at https://random.dog/woof.json?ref=apilist.fun.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes