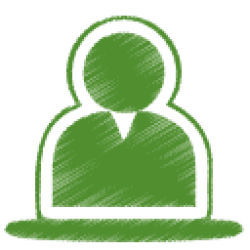
RandomUser
DevelopmentExploring the Randomuser API with JavaScript
Randomuser is a fantastic API that provides developers with a way of generating random user data. This API can be used in many different ways, making it a great tool for developers to use.
To get started with the Randomuser API, you'll first need to make a GET request to the API endpoint. The endpoint is https://randomuser.me/api, and it accepts several different parameters to specify what type of data you want to receive.
Let's take a look at some example code in JavaScript to see how we can use the Randomuser API.
Basic Request
The following code makes a basic request to the API and logs the results to the console:
fetch('https://randomuser.me/api/')
.then(response => response.json())
.then(data => console.log(data));
This code uses the fetch()
function to make a GET request to the API endpoint. The response
object is then converted to a JSON object using the json()
method, and the resulting data is logged to the console.
Filtering Results
You can also filter the results of your request by specifying various parameters. For example, you can limit the number of results by setting the results
parameter to a specific number. Here's an example of how to request only one result:
fetch('https://randomuser.me/api/?results=1')
.then(response => response.json())
.then(data => console.log(data));
Using Promises and Async/Await
When working with APIs, it's common to use promises to handle asynchronous data. Here's an example of how you can use Promises with the Randomuser API:
const getRandomUser = () => {
return new Promise((resolve, reject) => {
fetch('https://randomuser.me/api/')
.then(response => response.json())
.then(data => resolve(data))
.catch(error => reject(error));
});
};
getRandomUser()
.then(data => console.log(data))
.catch(error => console.error(error));
If you're using ECMAScript 2017 or later, you can also take advantage of async/await to handle asynchronous data. Here's an example:
const getRandomUser = async () => {
try {
const response = await fetch('https://randomuser.me/api/');
const data = await response.json();
return data;
} catch (error) {
console.error(error);
}
};
getRandomUser().then(data => console.log(data));
Conclusion
Using the Randomuser API is easy to do with JavaScript. With just a few lines of code, you can create some fun projects that generate random user data. Be sure to check out the Randomuser API documentation to learn more about all the different parameters you can use!