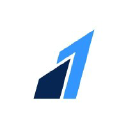
Razorpay IFSC
FinanceUnveil the world of financial operations with the Indian Financial Systems Code (IFSC) API. This phenomenal tool provides you with the flexibility to fetch bank branch codes across India, bridging the gap between conventional banking systems and modern digital operations. Its seamless integration accelerates traditional banking workflows, encourage frictionless access to data, and supports strategic decision-making processes in financial service applications. You can find comprehensive documentation available on the Razorpay website.
Our IFSC API not only takes security to the next level but also ensures efficient management of sensitive banking data. It harnesses the potential to revolutionize the way you carry out your financial transactions, transforming business operations immensely. With the IFSC API, you can now bid farewell to the hassles of manual data inputs, errors, and unnecessary delays.
Benefits of using this API:
- Digitally enabled access to bank branch codes across India.
- Accelerates banking operations and reduces operational inefficiencies.
- Drastically reduces manual data entry and the associated errors.
- Ensures secure and reliable data handling adhering to industry standards.
- Promotes efficient decision-making in financial service applications.
Here is an example of how to call this API using JavaScript:
var request = require('request');
var options = {
'method': 'GET',
'url': 'https://api.razorpay.com/v1/ifsc/BANKBRANCHID', //Replace with the actual bank branch ID
'headers': {
'Content-Type': 'application/json'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
This is a simple GET request to the API endpoint, replace 'BANKBRANCHID' with the actual bank branch ID you are searching for. The response will contain the bank codes and related information.