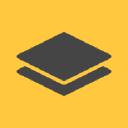
Remove.bg
PhotographyThe Image Background Removal API offers an effortless solution for users looking to eliminate backgrounds from images quickly and accurately. This powerful tool caters to a wide range of applications, from enhancing product photos for e-commerce platforms to creating visually appealing graphics for social media. By leveraging advanced algorithms, the API ensures high-quality results that maintain the integrity of the original subject while seamlessly removing unwanted backgrounds. With an easy-to-use interface and flexible integration options, developers can incorporate this API into their applications, providing users with enhanced editing capabilities and a streamlined workflow.
Using the Image Background Removal API presents numerous advantages, such as:
- Time Efficiency: Rapid processing and instant results save users valuable time.
- Cost-Effective: Eliminates the need for expensive graphic design software and services.
- User-Friendly: Simple API calls make it accessible for developers of all skill levels.
- High-Quality Outputs: Leverages state-of-the-art technology for superior image editing results.
- Scalability: Easily handles varying volumes of image processing, making it a great choice for businesses of all sizes.
Here’s a JavaScript code example demonstrating how to call the API:
const axios = require('axios');
const fs = require('fs');
const removeBgFromImage = async (imagePath) => {
const apiKey = 'YOUR_API_KEY'; // Replace with your API key
const url = 'https://api.remove.bg/v1.0/removebg';
const formData = new FormData();
formData.append('image_file', fs.createReadStream(imagePath));
formData.append('size', 'auto');
try {
const response = await axios.post(url, formData, {
headers: {
'X-Api-Key': apiKey,
...formData.getHeaders(),
},
responseType: 'arraybuffer',
});
fs.writeFileSync('output.png', response.data);
console.log('Background removed and saved as output.png');
} catch (error) {
console.error('Error removing background:', error);
}
};
removeBgFromImage('path/to/your/image.jpg');