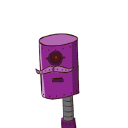
RoboHash
DevelopmentGenerate Unique Robot Avatars using RoboHash API
Are you looking for a free robot avatar generator API? Look no further than RoboHash!
RoboHash is a API that generates unique robot avatars based on a provided input string. It's a great way to add visual identity to your application or project.
Using the RoboHash API is an easy process. Here are a few examples using JavaScript:
Example #1: Generate Robot Avatar Image
To generate a robot avatar image, use fetch
method and specify the input string after https://robohash.org/
.
const input = 'example';
const img = document.createElement('img');
const url = `https://robohash.org/${input}?size=200x200`;
fetch(url)
.then(response => response.blob())
.then(blob => {
img.src = URL.createObjectURL(blob);
document.body.appendChild(img);
});
Example #2: Generate Robot Avatar Image with Custom Options
The RoboHash API allows you to add custom options to the avatar. Here's how you can use custom options to create your own unique avatars:
const options = {
size: '400x400',
set: 'set1',
bgset: 'bg1',
format: 'svg',
};
const input = 'example';
const img = document.createElement('img');
const queryParams = new URLSearchParams(options).toString();
const url = `https://robohash.org/${input}?${queryParams}`;
fetch(url)
.then(response => response.blob())
.then(blob => {
img.src = URL.createObjectURL(blob);
document.body.appendChild(img);
});
Example #3: Generate Robot Avatar Image with Gravatar Integration
If you want to integrate your RoboHash API calls with Gravatar, you can specify the Gravatar email instead of the input string:
const options = {
size: '200x200',
};
const gravatarEmail = 'useremail@example.com';
const img = document.createElement('img');
const queryParams = new URLSearchParams(options).toString();
const url = `https://robohash.org/${gravatarEmail}?${queryParams}`;
fetch(url)
.then(response => response.blob())
.then(blob => {
img.src = URL.createObjectURL(blob);
document.body.appendChild(img);
});
That's it! You can now start experimenting with RoboHash API and create unique robot avatars for your application or project.