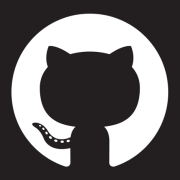
Ron Swanson Quoutes
MediaAccessing Ron Swanson Quote API with JavaScript
If you're a Parks and Recreation fan, you're probably familiar with the character Ron Swanson. Did you know that there's a public API that allows you to retrieve random Ron Swanson quotes? In this blog post, we'll explore how to access this API using JavaScript.
Getting Started
First, let's take a look at the API documentation. The documentation specifies that we can retrieve quotes by sending a GET request to the following endpoint:
https://ron-swanson-quotes.herokuapp.com/v2/quotes
The response from the API will be an array of strings, each containing a Ron Swanson quote.
Making Requests with JavaScript
To retrieve data from the API, we can use the fetch
method, which allows us to send HTTP requests and receive responses.
fetch('https://ron-swanson-quotes.herokuapp.com/v2/quotes')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In the above code, we first send a GET
request to the API endpoint using the fetch
method. Once we receive a response, we extract the JSON data using the json()
method. We then log the data to the console, or catch any errors that might occur.
Using the Data
Once we have the data, we can use it to display a random Ron Swanson quote on our website. One way to do this is to generate a random index and use that to access a quote from the array.
const displayQuote = () => {
fetch('https://ron-swanson-quotes.herokuapp.com/v2/quotes')
.then(response => response.json())
.then(data => {
const randomIndex = Math.floor(Math.random() * data.length);
const quote = data[randomIndex];
document.getElementById('quote').innerText = quote;
})
.catch(error => {
console.error(error);
});
};
In the example above, we define a function displayQuote()
that sends a GET
request to the API and retrieves a random quote. We first generate a random index between 0 and the length of the array using the Math.random()
method. We then use this index to access a quote from the array, and set the inner text of an HTML element with the ID of quote
to the quote.
Conclusion
In this blog post, we've explored how to send requests to the Ron Swanson Quote API using JavaScript. We've also shown how to use the retrieved data to display a random Ron Swanson quote on a website. Give it a try and see what kind of hilarious quotes you can come up with!