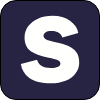
Scanii
Anti-MalwareThe Simple REST API is an efficient solution designed to scan documents and files for potential threats, ensuring that your data remains secure. With its easy-to-use interface, developers can integrate powerful threat detection functionalities into their applications seamlessly. This API is capable of processing various document formats, making it a versatile tool for businesses looking to safeguard their information from malware and other security risks. By leveraging advanced scanning technology, users can receive quick feedback on the safety of their documents, allowing for proactive security measures.
Utilizing this API comes with numerous benefits that enhance the security and operational efficiency of your applications. Key advantages include:
- Rapid document scanning to reduce potential downtime
- Comprehensive threat detection that covers a wide range of file types
- Easy integration into existing systems, supporting various programming languages
- Detailed reporting on scanned files, providing insights into detected threats
- Scalable solution that caters to both small and large enterprises
Here’s an example of how to call the API using JavaScript:
const fetch = require('node-fetch');
async function scanDocument(file) {
const apiUrl = 'https://api.scanii.com/scan';
const formData = new FormData();
formData.append('file', file);
const response = await fetch(apiUrl, {
method: 'POST',
body: formData,
headers: {
'Authorization': 'Bearer YOUR_API_KEY',
},
});
if (response.ok) {
const jsonResponse = await response.json();
console.log('Scan Result:', jsonResponse);
} else {
console.error('Error scanning document:', response.statusText);
}
}
// Usage example
const fileInput = document.querySelector('input[type="file"]');
fileInput.addEventListener('change', (event) => {
const file = event.target.files[0];
if (file) {
scanDocument(file);
}
});