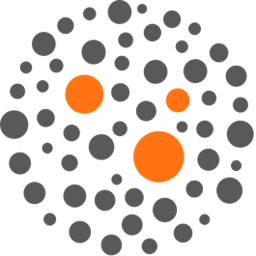
Schiphol Airport
TransportationExploring the Schiphol API using JavaScript
Are you looking to integrate Schiphol airport data into your web application, or just want to explore the airport's public API? Look no further than https://developer.schiphol.nl/
. In this blog post, we will cover how to use the Schiphol API with JavaScript, and provide a few example API calls.
Getting Started
First, make sure that you have an account on the Schiphol Developer website. You will need to create an application in order to receive an API key. Once you have an API key, you can start making requests to the Schiphol API.
We will be using the fetch
method to make API calls. This method is available in most modern browsers, and allows us to easily fetch data from a remote server.
Example API Calls
Get All Airlines
To get a list of all airlines that operate at Schiphol, we can use the https://api.schiphol.nl/public-flights/airlines
API endpoint. Here is an example of how to fetch this data using JavaScript:
fetch('https://api.schiphol.nl/public-flights/airlines', {
headers: {
'app_id': '<YOUR_APP_ID>',
'app_key': '<YOUR_APP_KEY>'
}
})
.then(response => response.json())
.then(data => console.log(data.airlines))
.catch(error => console.error(error));
Replace <YOUR_APP_ID>
and <YOUR_APP_KEY>
with your Schiphol Developer website credentials.
Get Flight Information
To get real-time information about a flight, we can use the https://api.schiphol.nl/public-flights/flights
API endpoint and specify the flight number. Here is an example of how to fetch data about the flight numbered KL1234 using JavaScript:
fetch('https://api.schiphol.nl/public-flights/flights/KL1234', {
headers: {
'app_id': '<YOUR_APP_ID>',
'app_key': '<YOUR_APP_KEY>'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Get Flight Schedule
To get the schedule of a flight, we can use the https://api.schiphol.nl/public-flights/flights/${flightNumber}/schedule
API endpoint. Here is an example of how to fetch the schedule of the flight numbered KL1234 using JavaScript:
fetch('https://api.schiphol.nl/public-flights/flights/KL1234/schedule', {
headers: {
'app_id': '<YOUR_APP_ID>',
'app_key': '<YOUR_APP_KEY>'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
In this blog post, we have covered how to use the Schiphol API with JavaScript. We have provided code examples for a few different types of API calls, but there are many more endpoints available to explore. If you are interested in diving deeper, be sure to check out the Schiphol Developer website for more information. Happy coding!