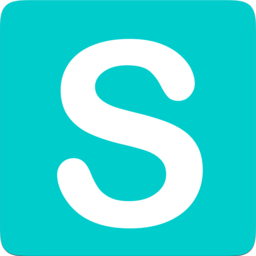
ScraperApi
DevelopmentMaking Data Scraping Easier with ScraperAPI
Data scraping is an essential process for businesses and individuals alike who want to access information from websites for various purposes. However, it can be challenging to perform data scraping tasks due to the complex nature of many modern websites. ScraperAPI offers a solution for this problem by providing a simple to use API that allows you to retrieve data from any website.
Here is a brief guide on how to use ScraperAPI in your JavaScript code to perform various data scraping tasks.
Setting up the API
Before you can start using ScraperAPI, you need to sign up and get an API key from their website. Once you have the API key, you can start making requests to the ScraperAPI endpoint.
Retrieving Data from a Website
To retrieve data from a website using ScraperAPI, you need to make an HTTP GET request to the ScraperAPI endpoint, passing the website URL as a parameter. Here's an example code of how to achieve this in JavaScript:
const ScraperAPI = require('scraperapi-sdk');
const client = new ScraperAPI({ apiKey: 'YOUR_API_KEY' });
client.get('http://example.com/').then(({ data }) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
In the above example, we create an instance of the ScraperAPI client, passing our API key as an option. We then make an HTTP GET request to retrieve the website data, and finally, we log the data to the console.
Passing Additional Parameters
You can pass additional parameters to the ScraperAPI endpoint to modify the behavior of your data retrieval. Here's an example code that adds extra parameters:
const ScraperAPI = require('scraperapi-sdk');
const client = new ScraperAPI({ apiKey: 'YOUR_API_KEY' });
const options = {
country_code: 'us',
premium: 'true',
render: 'true'
};
client.get('http://example.com/', options).then(({ data }) => {
console.log(data);
}).catch((error) => {
console.log(error);
});
In this example, we pass additional parameters, such as the country code, premium status, and rendering option, to the endpoint through the second argument of the get
method. These parameters will help you customize the way data is retrieved in a more precise way.
Conclusion
ScraperAPI is an excellent API that can help you perform data scraping tasks with ease. With its simple integration and useful features, it provides a significant advantage when working with data from websites. By following these examples in your JavaScript code, you can start using ScraperAPI in your projects today!