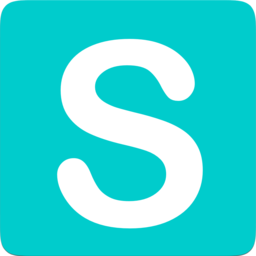
Scraper API
DevelopmentScraper API handles proxies, browsers, and CAPTCHAs, so you can get the HTML from any web page with a simple API call!
π Documentation & Examples
Everything you need to integrate with Scraper API
π Quick Start Examples
// Scraper API API Example
const response = await fetch('https://www.scraperapi.com/documentation', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Introduction to Scraper API
Scraper API is a powerful tool that enables developers to easily automate web scraping tasks by providing a fast, reliable, and fully managed proxy service. With its unique features and advanced functionality, Scraper API is quickly becoming a popular choice among developers looking to build high-quality web scraping applications.
In this blog post, we will explore some of the key features and use cases of Scraper API and provide some sample JavaScript code to demonstrate how easily you can integrate it into your own applications.
Getting started with Scraper API
To get started with Scraper API, you will first need to create an account and obtain an API key. You can do this by visiting the Scraper API website and following the instructions provided.
Once you have your API key, you can start using Scraper API to fetch webpages and process the data. Here is a simple example in JavaScript that shows how to fetch a webpage using Scraper API:
const apiKey = 'YOUR_API_KEY'
const url = 'https://example.com'
const params = {
api_key: apiKey,
url: url
}
fetch(`https://api.scraperapi.com?${new URLSearchParams(params)}`)
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.error(error))
In this example, we are passing our API key and the URL of the webpage we want to scrape to the Scraper API endpoint. The fetch function is used to make a request to the Scraper API endpoint and retrieve the HTML content of the webpage.
Advanced usage of Scraper API
Scraper API provides a number of advanced features that enable developers to build more complex and sophisticated web scraping applications. Here are some examples of how to use these features in JavaScript:
Location-based web scraping
Scraper API supports location-based scraping, which allows you to specify the location where you want your requests to originate from. Here is an example in JavaScript that demonstrates how to use the location-based scraping feature:
const apiKey = 'YOUR_API_KEY'
const url = 'https://example.com'
const params = {
api_key: apiKey,
url: url,
country_code: 'US'
}
fetch(`https://api.scraperapi.com?${new URLSearchParams(params)}`)
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.error(error))
In this example, we are specifying the country code as 'US', which means that our request will originate from the United States. This can be useful if you want to scrape data that is only available in certain regions.
Pagination and limit handling
Scraper API also has built-in support for pagination and limit handling, which makes it easy to scrape large amounts of data from websites. Here is an example in JavaScript that shows how to use the pagination and limit handling feature:
const apiKey = 'YOUR_API_KEY'
const url = 'https://example.com'
const params = {
api_key: apiKey,
url: url,
page: 2,
limit: 50
}
fetch(`https://api.scraperapi.com?${new URLSearchParams(params)}`)
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.error(error))
In this example, we are specifying the page number as 2 and the limit as 50, which means that we want to retrieve the data from the second page of the website with a maximum of 50 items per page.
Conclusion
Scraper API is an incredibly powerful and useful tool that can help you automate web scraping tasks easily. In this blog post, we explored some of the key features and use cases of Scraper API and provided some sample JavaScript code to demonstrate how easily you can integrate it into your own applications. With its advanced functionality and ease of use, Scraper API is a must-have tool for any developer who needs to extract data from websites.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes