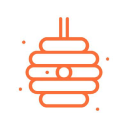
Scraping Ninja
DevelopmentScrapingNinja API Documentation
ScrapingNinja is a web scraping API that allows users to scrape data from websites quickly and efficiently. This documentation will guide you through the different methods and endpoints provided by the ScrapingNinja API.
Getting Started
Before you can start using the ScrapingNinja API, you need to sign up for an account on the ScrapingNinja website. Once you've created an account, you should receive an API key that you can use to authenticate your requests.
API Endpoints
GET /scrape
The /scrape
endpoint is used to scrape data from a website. You must include the website URL in the request parameters.
Example code in JavaScript:
const requestOptions = {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'api-key': YOUR_API_KEY,
}
}
const url = 'https://www.scrapingninja.co/scrape?website=WEBSITE_URL';
const response = await fetch(url, requestOptions);
const data = await response.json();
GET /scrape/proxy
The /scrape/proxy
endpoint is similar to the /scrape
endpoint, but it also uses a proxy to make the request. This can be useful if the website you're trying to scrape blocks requests from certain IP addresses.
Example code in JavaScript:
const requestOptions = {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'api-key': YOUR_API_KEY,
}
}
const url = 'https://www.scrapingninja.co/scrape/proxy?website=WEBSITE_URL&proxy=PROXY_URL';
const response = await fetch(url, requestOptions);
const data = await response.json();
GET /scrape/multi
The /scrape/multi
endpoint allows you to scrape multiple websites at once. You must include an array of website URLs in the request parameters.
Example code in JavaScript:
const requestOptions = {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'api-key': YOUR_API_KEY,
}
}
const websites = ['WEBSITE_URL_1', 'WEBSITE_URL_2', 'WEBSITE_URL_3'];
const url = `https://www.scrapingninja.co/scrape/multi?websites=${JSON.stringify(websites)}`;
const response = await fetch(url, requestOptions);
const data = await response.json();
GET /scrape/multi-proxy
The /scrape/multi-proxy
endpoint is similar to the /scrape/multi
endpoint, but it uses a proxy for each request.
Example code in JavaScript:
const requestOptions = {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'api-key': YOUR_API_KEY,
}
}
const websites = ['WEBSITE_URL_1', 'WEBSITE_URL_2', 'WEBSITE_URL_3'];
const proxies = ['PROXY_URL_1', 'PROXY_URL_2', 'PROXY_URL_3'];
const url = `https://www.scrapingninja.co/scrape/multi-proxy?websites=${JSON.stringify(websites)}&proxies=${JSON.stringify(proxies)}`;
const response = await fetch(url, requestOptions);
const data = await response.json();
Conclusion
ScrapingNinja provides a simple, easy-to-use API for web scraping. In this documentation, we've gone over the different endpoints provided by the API and included example code in JavaScript to demonstrate how to use them. If you have any questions or issues, you can contact the ScrapingNinja support team for assistance. Happy scraping!