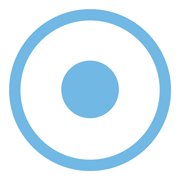
Screencast O Matic API
DevelopmentExploring Screencast-O-Matic Public API Docs
Screencast-O-Matic is a screen recording and video editing tool that allows users to create and share video content. With the Screencast-O-Matic public API, developers can access the platform's features to integrate them into their own web or mobile applications.
Getting Started with Screencast-O-Matic API
To begin using the Screencast-O-Matic API, you first need to sign-up for a developer account on their developers' website. Once you have registered, you can obtain your unique Access Key and Secret Key from your API dashboard.
With the credentials at hand, you can start making HTTP requests to the API endpoints to retrieve information or perform certain actions.
Retrieving Video Information
One of the main features of Screencast-O-Matic API is the ability to retrieve information about existing videos. This can be done by making a GET request to the videos/:id
endpoint, where id
refers to the unique ID of the video.
const videoId = '12345';
const accessKey = 'your-access-key';
const secretKey = 'your-secret-key';
fetch(`https://api.screencast-o-matic.com/v1/videos/${videoId}`, {
headers: {
'Authorization': `Basic ${btoa(`${accessKey}:${secretKey}`)}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we use JavaScript's fetch
function to make a GET request to the API endpoint for a video with ID 12345
. We pass our Access Key and Secret Key as the Authorization
header with the request.
Embedding Videos in Web Page
Developers can also embed Screencast-O-Matic videos directly into their web pages. The API provides an endpoint that returns an HTML iframe
element with the video player. To use the endpoint, make a GET request to the videos/:id/player
endpoint.
const videoId = '12345';
fetch(`https://api.screencast-o-matic.com/v1/videos/${videoId}/player`)
.then(response => response.text())
.then(html => document.getElementById('player-container').innerHTML = html)
.catch(error => console.error(error));
In this example, we make a GET request to the videos/:id/player
endpoint and retrieve the HTML code for the embedded video player. We then insert this HTML code into a container element in the front-end HTML page with id="player-container"
.
Conclusion
The Screencast-O-Matic public API provides developers with a powerful tool to access and integrate platform features into their web or mobile applications. By following the documentation and examples provided, developers can create new, innovative video experiences and workflows.