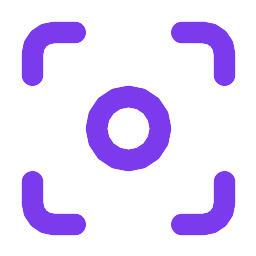
ScreenshotOne
DevelopmentSimplify Screenshot Rendering with a Single API Call
Rendering screenshots has never been easier! With our API, you can eliminate the complexities of managing browser clusters and handling countless edge cases. This streamlined approach lets you focus on your core tasks while we take care of the rest.
By using this API, you gain a powerful solution that saves time, reduces overhead, and ensures reliable results. Simplify your workflow and elevate your efficiency today.
5 Benefits of Using This API
- Ease of Use: Render screenshots with just one API call.
- No Infrastructure Management: Avoid the hassle of managing browser clusters.
- Edge Case Handling: Automatically handle complex corner cases.
- Scalable Solution: Easily scale without worrying about infrastructure limits.
- Cost Efficiency: Save on operational costs by leveraging a streamlined service.
Example Code in JavaScript
const axios = require('axios');
const apiKey = 'your-api-key'; // Replace with your actual API key
const url = 'https://example.com'; // Replace with the URL you want to capture
(async () => {
try {
const response = await axios.get('https://screenshotone.com/api', {
params: {
url: url,
access_key: apiKey
}
});
console.log('Screenshot URL:', response.data.screenshot_url);
} catch (error) {
console.error('Error rendering screenshot:', error);
}
})();