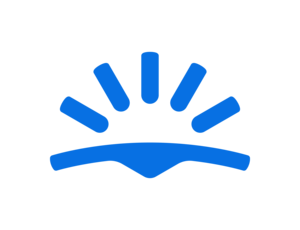
Skyscanner Travel API
TransportationSkyScanner API: Your Travel Companion
SkyScanner is a travel website that helps users search for and compare prices on flights, hotels, and car rentals. The website provides an API to its partners to allow them to integrate SkyScanner's services into their own applications.
Getting Started
To use SkyScanner's API, you will need to create an affiliate account and obtain an API key. You can do both of these by visiting the SkyScanner Partners website.
API Endpoints
SkyScanner offers several APIs for different travel-related services. These include:
- Flight Search API
- Hotel Search API
- Car Hire Search API
- Travel Insight API
All of these APIs use HTTPS requests and responses in JSON format. In this blog post, we will provide example code in JavaScript for the Flight Search API.
Flight Search API
The Flight Search API allows you to search for flights based on criteria such as origin, destination, departure date, and return date. Here is an example JavaScript code for making a flight search:
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://partners.api.skyscanner.net/apiservices/browsequotes/v1.0/GB/GBP/en-GB/EDI-sky/LHR-sky/2023-07-01/2023-07-31?apiKey=<YOUR_API_KEY>')
xhr.setRequestHeader("Content-type", "application/json");
xhr.send();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
const response = JSON.parse(xhr.responseText);
console.log(response);
}
};
In the above code, we are making a GET request to the Flight Search API with the following parameters:
GB/GBP/en-GB
: The market, currency, and locale for the search resultsEDI-sky/LHR-sky
: The origin and destination airports in their respective IATA codes2023-07-01/2023-07-31
: The departure and return dates for the search results<YOUR_API_KEY>
: Your SkyScanner API key
Once we receive the response from the API, we are parsing it as JSON and logging it to the console. You can modify this code to display the search results on your application UI instead.
Conclusion
SkyScanner's API provides an easy way for travel businesses to offer their customers the convenience of searching for and booking flights, hotels, and car rentals. With the JavaScript example code provided in this blog post, you can get started using the Flight Search API yourself. Happy coding!