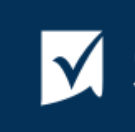
Smartsheet
BusinessExploring the Smartsheet API with JavaScript
If you're looking to integrate your application or website with Smartsheet, then their API documentation is a great place to start. You can find the Smartsheet API documentation at https://smartsheet.redoc.ly/.
In this post, we'll take a look at some of the API examples available on the site and explore how to use them with JavaScript.
Authentication
Before we start making API calls with JavaScript, we need to authenticate first. The Smartsheet API supports OAuth2 for authentication. You can learn more about Smartsheet OAuth2 in their official documentation.
For the purpose of this post, we'll use personal access tokens to authenticate our API requests. You can generate a new personal access token from your Smartsheet account settings.
Once you have a personal access token, you can use it to authenticate your requests by adding an Authorization header as follows:
const accessToken = 'YOUR_ACCESS_TOKEN';
const headers = {
'Authorization': `Bearer ${accessToken}`
};
Working with Sheets
The Smartsheet API allows you to perform many operations on your sheets, such as accessing the sheet data, modifying the sheet properties, and adding rows to the sheet.
Accessing Sheet Data
To retrieve the data of a specific sheet, you can make a GET request to the /sheets/{sheetId}
endpoint. The following example shows how to retrieve the data of a sheet with ID 123456789
:
const sheetId = '123456789';
fetch(`https://api.smartsheet.com/2.0/sheets/${sheetId}`, {
headers
})
.then(response => response.json())
.then(data => console.log(data));
Modifying Sheet Properties
To update the properties of a sheet, you can make a PUT request to the /sheets/{sheetId}
endpoint. The following example shows how to update the name of a sheet with ID 123456789
:
const sheetId = '123456789';
const name = 'New Sheet Name';
fetch(`https://api.smartsheet.com/2.0/sheets/${sheetId}`, {
method: 'PUT',
headers: {
...headers,
'Content-Type': 'application/json'
},
body: JSON.stringify({
name
})
})
.then(response => response.json())
.then(data => console.log(data));
Adding Rows to a Sheet
To add rows to a sheet, you can make a POST request to the /sheets/{sheetId}/rows
endpoint. The following example shows how to add a new row with data { Name: 'John', Age: 30 }
to a sheet with ID 123456789
:
const sheetId = '123456789';
const row = {
toBottom: true,
cells: [
{ columnId: 'name', value: 'John' },
{ columnId: 'age', value: 30 }
]
};
fetch(`https://api.smartsheet.com/2.0/sheets/${sheetId}/rows`, {
method: 'POST',
headers: {
...headers,
'Content-Type': 'application/json'
},
body: JSON.stringify([row])
})
.then(response => response.json())
.then(data => console.log(data));
Conclusion
In this post, we've explored some of the available Smartsheet API examples and demonstrated how to use them with JavaScript. Remember to always authenticate your API requests with either OAuth2 or personal access tokens.
If you're interested in learning more about the Smartsheet API, be sure to check out their official documentation.