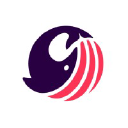
SonarQube
DevelopmentSonarQube REST APIs provide powerful tools for developers to automate the detection of bugs, code smells, and security vulnerabilities within their codebases. With these APIs, teams can seamlessly integrate SonarQube's advanced static code analysis into their CI/CD pipelines, ensuring that code quality is maintained throughout the development lifecycle. By utilizing these APIs, organizations gain the ability to perform comprehensive code assessments, identify potential pitfalls early in the development process, and foster a culture of continuous improvement in software quality. The extensive documentation available at SonarCloud API Documentation offers clear guidance on how to effectively implement these APIs in various programming environments.
Some advantages of using SonarQube REST APIs include:
- Automated detection of code quality issues, reducing manual inspections.
- Early identification of security vulnerabilities to mitigate risks.
- Easy integration into existing development workflows and tools.
- Enhanced collaboration among teams with actionable insights.
- Improved overall software quality and maintainability over time.
Here’s a JavaScript code example for calling the SonarQube REST API to retrieve project issues:
const axios = require('axios');
const SONARQUBE_URL = 'https://sonarcloud.io/api/issues/search';
const PROJECT_KEY = 'your_project_key';
const SONARQUBE_TOKEN = 'your_sonarcloud_token';
async function fetchProjectIssues() {
try {
const response = await axios.get(SONARQUBE_URL, {
params: {
componentKeys: PROJECT_KEY,
resolved: false,
types: 'BUG, CODE_SMELL, VULNERABILITY'
},
headers: {
'Authorization': `Basic ${Buffer.from(`${SONARQUBE_TOKEN}:`).toString('base64')}`
}
});
console.log('Project Issues:', response.data);
} catch (error) {
console.error('Error fetching project issues:', error);
}
}
fetchProjectIssues();