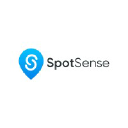
SpotSense
GeocodingThe SpotSense API offers a powerful solution for developers looking to enhance their mobile applications with location-based interactions. This innovative API enables seamless integration of geospatial data, allowing apps to unlock new functionality such as personalized content delivery, location tracking, and user engagement. With SpotSense, you can create immersive experiences that cater to the unique environment of your users, enabling them to interact with their surroundings in real-time. Whether you are developing a retail app that sends notifications based on user proximity to a store or a travel app that provides tailored suggestions based on location, SpotSense equips you with the tools to engage users effectively.
By leveraging the SpotSense API, developers can enjoy several key benefits:
- Enhanced User Engagement: Create memorable interactions that keep users coming back.
- Personalized Experiences: Tailor content and notifications based on user location.
- Real-Time Data Access: Receive immediate updates on user interactions and movements.
- Easy Integration: Simplify development with comprehensive documentation and support.
- Scalability: Effortlessly scale your application as user demand grows.
Here is a JavaScript code example to call the SpotSense API:
const axios = require('axios');
const SPOTSENSE_API_URL = 'https://api.spotsense.io/v1/interaction';
async function addLocationInteraction(userId, locationData) {
try {
const response = await axios.post(SPOTSENSE_API_URL, {
userId: userId,
location: locationData
});
console.log('Interaction recorded:', response.data);
} catch (error) {
console.error('Error recording interaction:', error);
}
}
// Example usage
const userId = 'user123';
const locationData = {
latitude: 37.7749,
longitude: -122.4194,
description: 'Visited the Golden Gate Park'
};
addLocationInteraction(userId, locationData);