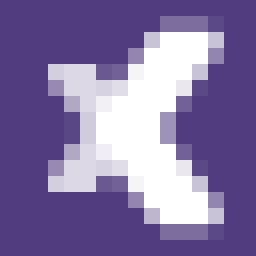
Sqlify API
Cloud Storage & File SharingExploring the Numidian API with JavaScript
Are you in need of an API that provides you with financial data? Look no further, as Numidian API is here to help. With a user-friendly API documentation, Numidian provides developers with access to global stock and forex pricing data that can help individuals make informed decisions as they buy and sell stocks.
To explore the Numidian API with JavaScript, let's take a look at some example codes that you can use.
Authentication
Before we begin, we must authenticate our connection to the API with the API key. Here is an example to set up the API key:
const API_KEY = 'place-your-api-key-here';
Endpoint: Stock quotes
To retrieve the latest stock quote, we can use the following code:
fetch(`https://api.numidian.io/api/quotes/stock/GOOGL?apikey=${API_KEY}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we fetch the stock quote for the company Alphabet Inc. (GOOGL). The response will be in JSON format, containing the current stock price, change percentage, and other relevant data.
Endpoint: Forex quotes
To retrieve the current forex rates, we can use the following code:
fetch(`https://api.numidian.io/api/quotes/forex?from_currency=USD&to_currency=EUR&apikey=${API_KEY}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we fetch the forex rate for USD to EUR. The response will be in JSON format, containing the current exchange rate, and the time of the update.
Endpoint: End of day data
To retrieve end of day stock prices, we can use the following code:
fetch(`https://api.numidian.io/api/historical/stock/GOOGL?apikey=${API_KEY}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we fetch the end of day stock prices for the company Alphabet Inc. (GOOGL) for the most recent trading day.
Conclusion
Numidian API provides users with a variety of financial data that can help investors make informed decisions about trading. With JavaScript, developers can easily retrieve the stock and forex quotes as well as end of day data. The possibilities are endless with Numidian API.