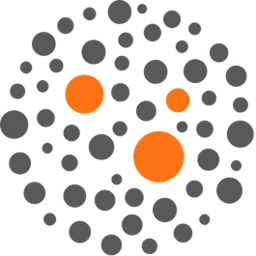
Post Nord
TransportationUsing PostNord Public APIs in JavaScript
PostNord provides a range of public APIs that developers can use to access shipping and logistics data. These APIs can be integrated into apps and websites, allowing users to access real-time information about shipments, pickup points, and delivery times.
In order to use the PostNord APIs in JavaScript, you will need to obtain an API key from the developer portal. Once you have your key, you can use it to make requests to the various endpoints provided by the APIs. Here are some examples of how to use the PostNord APIs in JavaScript:
Retrieving Shipping Information
To retrieve shipping information for a specific tracking number, you can use the shipment
endpoint. Here's an example of how to use this endpoint in JavaScript:
const apiKey = 'your-api-key';
const trackingNumber = 'your-tracking-number';
fetch(`https://api2.postnord.com/rest/shipment/v2/trackandtrace/findByIdentifier.json?id=${trackingNumber}&locale=en&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we are using the fetch()
method to make a GET request to the shipment
endpoint. We provide the tracking number as a query parameter, along with our API key and the desired format and locale. When the response is returned, we convert it to JSON and log it to the console.
Finding Pickup Points
To find pickup points near a specific location, you can use the pickuppoints
endpoint. Here's an example of how to use this endpoint in JavaScript:
const apiKey = 'your-api-key';
const latitude = 'your-latitude';
const longitude = 'your-longitude';
fetch(`https://api2.postnord.com/rest/businesslocation/v1/pickuppoints/nearby.json?latitude=${latitude}&longitude=${longitude}&maxNo=10&radius=10000&locale=en&origin=coord&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we are using the fetch()
method to make a GET request to the pickuppoints
endpoint. We provide the latitude and longitude as query parameters, along with other optional parameters such as the maximum number of results and the search radius. When the response is returned, we convert it to JSON and log it to the console.
Retrieving Delivery Times
To retrieve delivery times for a specific shipment, you can use the deliverytime
endpoint. Here's an example of how to use this endpoint in JavaScript:
const apiKey = 'your-api-key';
const fromPostalCode = 'your-from-postal-code';
const toPostalCode = 'your-to-postal-code';
fetch(`https://api2.postnord.com/rest/timeapi/v1/deliverytime.json?from=${fromPostalCode}&to=${toPostalCode}&weightInGrams=500&productCode=PA&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we are using the fetch()
method to make a GET request to the deliverytime
endpoint. We provide the postal codes for the origin and destination of the shipment, along with other optional parameters such as the weight of the shipment and the product code. When the response is returned, we convert it to JSON and log it to the console.
These are just a few examples of how to use the PostNord APIs in JavaScript. With these APIs, developers can create powerful logistics applications that make it easy for users to track shipments, find pickup points, and more.