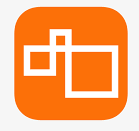
Swift Kanban
BusinessUsing the SwiftKanban API with JavaScript
The SwiftKanban API provides developers with a way to programmatically interact with SwiftKanban's web services. This can be useful for automating tasks or integrating SwiftKanban with other applications. In this blog post, we will explore how to use the SwiftKanban API with JavaScript and provide some example code.
Getting Started
To use the SwiftKanban API with JavaScript, you will need to obtain an API key from your SwiftKanban account. You can find instructions on how to do this in the API documentation. Once you have your API key, you can start making requests to the SwiftKanban API.
Making API Requests
To make a request to the SwiftKanban API with JavaScript, you will need to use the fetch
function. This is a built-in function in modern browsers that allows you to make HTTP requests. Here is an example of how to make a request to the SwiftKanban API to get a list of all the boards:
fetch('https://webapi.swiftkanban.com/api/boards', {
headers: {
'Authorization': 'Bearer <your-api-key>'
}
})
.then(response => response.json())
.then(data => console.log(data));
In this example, we are making a GET request to the https://webapi.swiftkanban.com/api/boards
endpoint and passing our API key in the Authorization
header. When the response is received, we are parsing the JSON data and logging it to the console.
Examples
Getting a Board
To get a board with a specific ID, you can make a GET request to the /api/boards/{boardid}/
endpoint. Here is an example:
const boardId = '<your-board-id>';
fetch(`https://webapi.swiftkanban.com/api/boards/${boardId}/`, {
headers: {
'Authorization': 'Bearer <your-api-key>'
}
})
.then(response => response.json())
.then(data => console.log(data));
Creating a Card
To create a card in a board, you can make a POST request to the /api/boardcards/
endpoint. Here is an example:
const boardId = '<your-board-id>';
const cardData = {
'title': 'New Card',
'description': 'This is a new card',
'swimlaneId': '<your-swimlane-id>',
'columnId': '<your-column-id>',
};
fetch(`https://webapi.swiftkanban.com/api/boardcards/`, {
method: 'POST',
headers: {
'Authorization': 'Bearer <your-api-key>',
'Content-Type': 'application/json'
},
body: JSON.stringify(cardData)
})
.then(response => response.json())
.then(data => console.log(data));
In this example, we are creating a new card with the title "New Card" and the description "This is a new card" in the swimlane and column specified by swimlaneId
and columnId
. The new card data is passed in the request body as a JSON string.
Updating a Card
To update a card in a board, you can make a PUT request to the /api/boardcards/{cardid}/
endpoint. Here is an example:
const cardId = '<your-card-id>';
const cardData = {
'title': 'Updated Card',
'description': 'This is an updated card',
};
fetch(`https://webapi.swiftkanban.com/api/boardcards/${cardId}`, {
method: 'PUT',
headers: {
'Authorization': 'Bearer <your-api-key>',
'Content-Type': 'application/json'
},
body: JSON.stringify(cardData)
})
.then(response => response.json())
.then(data => console.log(data));
In this example, we are updating the title and description of a card with the specified ID. The updated card data is passed in the request body as a JSON string.
Conclusion
In this blog post, we have explored how to use the SwiftKanban API with JavaScript and provided some example code for common use cases. With the SwiftKanban API, you can automate tasks and integrate SwiftKanban with other applications. If you have any questions or need help getting started with the SwiftKanban API, be sure to check out the API documentation.