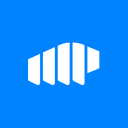
Tardigrade Object Storage
Cloud Storage & File SharingExploring the Public API Documentation of Tardigrade.io
If you're interested in using the public API provided by Tardigrade.io, you're in the right place. This API allows you to access various features of the cloud storage platform and make use of them in your own projects and applications. In this post, we'll take a closer look at the API documentation and explore some sample code using JavaScript.
Getting Started
Before jumping into the code, let's take a moment to review the basic concepts of the API. The Tardigrade.io API has four main endpoints:
- Bucket API: used for managing buckets
- Object API: used for managing objects within a bucket
- Download API: used for downloading objects
- Upload API: used for uploading objects
Each of these endpoints has specific methods and parameters that can be used to interact with the platform. You can find detailed documentation about these methods and their usage on the Tardigrade.io API Reference website.
Using the API with JavaScript
Now that we've got an understanding of the API, let's explore some sample code using JavaScript. In order to interact with the API, we will be using the storj
package. This package is an HTTP client for the Tardigrade.io API, and it provides an easy-to-use interface for accessing the platform.
Installing the Package
First, we need to install the package. You can do this by running the following command in your terminal:
npm install storj
Creating a Client
Once we've installed the package, we can create a client object that will allow us to interact with the API. Here's an example code snippet:
const storj = require('storj');
const client = new storj.Environment({
apikey: 'MY_API_KEY', // Replace with your own key
baseUrl: 'https://api.storj.io/api',
});
In this example, we're creating a new client object using the storj.Environment
constructor. We're passing in our API key and the base URL for the API endpoint. Replace MY_API_KEY
with your own key.
Interacting with the API
Now that we have a client object, we can use it to interact with the various endpoints of the API. Here's an example of how to create a new bucket using the API:
client.createBucket('my_new_bucket', (err, bucket) => {
if (err) {
console.error(err);
} else {
console.log(bucket);
}
});
In this code, we're using the createBucket
method of the client object to create a new bucket. We're passing in the name of the new bucket ('my_new_bucket') as the first parameter, and a callback function that will be called when the operation is complete. If an error occurs, we'll log it to the console. If the operation is successful, we'll log the new bucket object to the console.
Additional Examples
Here are a few more examples of using the Tardigrade.io API with JavaScript:
// List all buckets
client.getBuckets((err, buckets) => {
if (err) {
console.error(err);
} else {
console.log(buckets);
}
});
// Upload a file to a bucket
const fileData = Buffer.from('Hello World!');
const uploadOptions = {
bucket: 'my_bucket',
uploadPath: '/hello.txt',
noProgress: true,
};
const upload = client.upload(fileData, uploadOptions);
upload.on('error', (err) => console.error(err));
upload.on('complete', () => console.log('Upload complete!'));
// Download a file from a bucket
const downloadOptions = {
bucket: 'my_bucket',
downloadPath: '/hello.txt',
};
const download = client.download(downloadOptions);
download.on('error', (err) => console.error(err));
download.on('complete', () => console.log('Download complete!'));
download.on('data', (data) => console.log(data));
These examples should give you a good idea of how to use the Tardigrade.io API with JavaScript. There are many more methods and parameters available, so be sure to check out the API Reference website for more information. Happy coding!