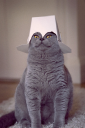
The Cat API
AnimalsIntroduction to the TheCatAPI
The TheCatAPI is a public API service that allows developers to access a vast collection of images and information relating to domestic cats. The API offers a variety of endpoints that can be accessed via HTTP requests, and returns data in a variety of formats including JSON, XML, and GIF.
The API provides developers with a wide range of options for customizing their requests, including various search parameters and filters, allowing for greater flexibility in the results returned by the API.
Using TheCatAPI
To start using TheCatAPI, you will first need to create an account and obtain an API key. Once you have an API key, you can start making requests to the API via HTTP.
Example Code
Installing Required Packages
Before we start making API requests, we need to install the required packages. In this example, we will be using Node.js and the request
package. You can install the request
package using the following command:
npm install request --save
Sending Requests to TheCatAPI
The following example shows how to make a request to the TheCatAPI to retrieve a random image of a cat using JavaScript:
const request = require('request');
const API_KEY = 'your_api_key_here';
const url = `https://api.thecatapi.com/v1/images/search?api_key=${API_KEY}`;
request.get(url, (error, response, body) => {
if (error) {
console.error(error);
return;
}
const data = JSON.parse(body)[0];
console.log(`Image URL: ${data.url}`);
});
In this example, we create a new request using the request
package and pass in the API endpoint URL with our API key. We then make a GET request to this URL, which returns a response object containing the requested data.
The response body is returned as a string, so we use the JSON.parse()
method to convert it into a JavaScript object. We then extract the URL of the first image returned from the API and log it to the console.
Searching for Cats
The following example shows how to make a request to the TheCatAPI to search for a specific breed of cat:
const request = require('request');
const API_KEY = 'your_api_key_here';
const breed = 'sphynx';
const url = `https://api.thecatapi.com/v1/breeds/search?api_key=${API_KEY}&q=${breed}`;
request.get(url, (error, response, body) => {
if (error) {
console.error(error);
return;
}
const data = JSON.parse(body);
console.log(`Breed Name: ${data[0].name}`);
console.log(`Origin: ${data[0].origin}`);
});
In this example, we create a new request to the /breeds
endpoint of the API, passing in our API key and the search query parameter q
with the value of the breed we want to search for.
We make a GET request to this URL, which returns a response object containing the requested data. We extract the name and origin of the breed from the first object in the returned data array and log it to the console.
Conclusion
The TheCatAPI offers developers a powerful tool for accessing a vast collection of cat-related data and images. By using the API, developers can easily retrieve information on a wide variety of topics relating to domestic cats, from breed information to image collections.