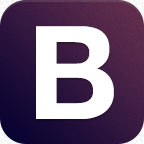
TheMealDB
Food & DrinkExploring TheMealDB API using JavaScript
Are you a food enthusiast who wants to explore different recipes and cuisines? Look no further than TheMealDB API! This public API offers a diverse collection of recipes, ingredients, categories, and more.
To get started, here are some examples of how to use TheMealDB API in JavaScript.
1. Getting all categories
fetch('https://www.themealdb.com/api/json/v1/1/categories.php')
.then(response => response.json())
.then(data => console.log(data.categories))
.catch(err => console.error(err));
This code sends a request to the API endpoint that returns all available categories. The response is then parsed into JSON and logged to the console.
2. Searching for meals by name
const keyword = 'chicken';
fetch(`https://www.themealdb.com/api/json/v1/1/search.php?s=${keyword}`)
.then(response => response.json())
.then(data => console.log(data.meals))
.catch(err => console.error(err));
In this example, we're fetching all meals from the API in which the name contains the keyword chicken
. The search term is passed as a query parameter in the API endpoint.
3. Getting a random meal
fetch('https://www.themealdb.com/api/json/v1/1/random.php')
.then(response => response.json())
.then(data => console.log(data.meals[0]))
.catch(err => console.error(err));
With this code, we can fetch a random meal from the API. The response object contains an array of one meal, which is then logged to the console.
4. Getting meal details by ID
const mealId = '52772';
fetch(`https://www.themealdb.com/api/json/v1/1/lookup.php?i=${mealId}`)
.then(response => response.json())
.then(data => console.log(data.meals[0]))
.catch(err => console.error(err));
This example shows how to get the details of a particular meal using its ID. We're fetching the meal whose ID is 52772
and then logging its details to the console.
5. Getting meals by ingredient
const ingredient = 'chicken';
fetch(`https://www.themealdb.com/api/json/v1/1/filter.php?i=${ingredient}`)
.then(response => response.json())
.then(data => console.log(data.meals))
.catch(err => console.error(err));
Finally, we have an example of fetching meals by their ingredient. In this case, we're fetching all meals that include the ingredient chicken
. The response object contains an array of meals that are then logged to the console.
These API examples demonstrate the vast amount of data that you can access from the TheMealDB API. Hopefully this inspires some creative recipe discovery for you. Happy cooking!