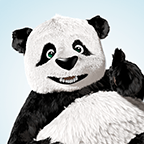
TinyPNG API
DevelopmentHow to Use TinyPNG API in JavaScript
TinyPNG is a popular image compression tool that can help reduce the size of your images without compromising on the image quality. The TinyPNG API extends the functionality of the TinyPNG service, making it possible for developers to integrate the tool into their own applications. In this article, we will take a look at how you can use the TinyPNG API in JavaScript.
Getting Started with the TinyPNG API
Before you can use the TinyPNG API, you will need to sign up for an API key. You can do this by visiting the TinyPNG Developers website at https://tinypng.com/developers
. Once you have an API key, you can use it to make API calls.
Installing the TinyPNG API for JavaScript
The TinyPNG API for JavaScript can be installed using npm. To install the package, run the following command:
npm install tinify --save
Using the TinyPNG API in JavaScript
To use the TinyPNG API in JavaScript, you will need to create an instance of the tinify
object. Once you have the object, you can use it to compress your images.
Here is an example of how to use the TinyPNG API in JavaScript:
const tinify = require("tinify");
tinify.key = "YOUR_API_KEY";
const source = tinify.fromFile("example.png");
source.toFile("example-compressed.png")
In this example, we first import the tinify
package and set the API key. Next, we load the image using the fromFile
method and then compress the image using the toFile
method.
Using the TinyPNG API with Node.js
To use the TinyPNG API with Node.js, you can use the fs
module to read and write image files. Here is an example:
const tinify = require("tinify");
const fs = require("fs");
tinify.key = "YOUR_API_KEY";
const source = tinify.fromBuffer(fs.readFileSync("example.png"));
source.toBuffer((err, resultData) => {
if (err) throw err;
fs.writeFileSync("example-compressed.png", resultData);
});
In this example, we first load the tinify
and fs
packages and set the API key. Next, we read the image file using the readFileSync
method and then compress the image using the toBuffer
method. Finally, we write the compressed image to file using the writeFileSync
method.
Conclusion
In this article, we have looked at how to use the TinyPNG API in JavaScript. We have covered how to sign up for an API key, install the package, and use the API with both Node.js and in the browser. With the TinyPNG API, you can improve the performance of your applications by compressing images and reducing their file size.