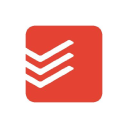
Todoist
Documents & ProductivityBuild your own system with todos data and access with their public APIs
📚 Documentation & Examples
Everything you need to integrate with Todoist
🚀 Quick Start Examples
// Todoist API Example
const response = await fetch('https://developer.todoist.com', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Discover the Power of Todoist API with JavaScript
Todoist is a popular tool for task management and to-do lists. With the Todoist API, you can connect the task-list capabilities of Todoist with your own applications. If you are a developer, you can integrate the Todoist API into your web or mobile applications.
In this article, we will walk through some of the examples of how to use the Todoist API in JavaScript. Before moving on, there are a few things you should know:
- To use the Todoist API, you need a valid API key. You can obtain the API key by visiting the developer section of Todoist and signing up for an account.
- Once you have the API key, you can use the API documentation to access the API endpoints and perform the desired functions.
- You can use various JavaScript libraries to perform API calls. For this article, we will use the popular "Axios" library.
Now, let's dive into the examples!
Example 1: Create a New Project
To create a new project in Todoist using the API in JavaScript, you need to send a POST request to the "/projects" endpoint. Here's how:
const axios = require('axios');
// create a new project
async function createNewProject(apiKey, projectName) {
try {
const response = await axios.post(
'https://api.todoist.com/rest/v1/projects',
{
name: projectName,
},
{
headers: {
Authorization: `Bearer ${apiKey}`,
},
}
);
return response.data;
} catch (error) {
console.error(error);
return null;
}
}
// usage
createNewProject('YOUR_API_KEY_HERE', 'My New Project');
The "createNewProject" function sends a POST request to the "/projects" endpoint with the project name in the request body. The API key is included in the request headers. If the request is successful, the function returns the response data, which includes the project ID.
Example 2: Get All Tasks
To get all tasks in a project, you need to send a GET request to the "/tasks" endpoint with a project ID. Here's how:
const axios = require('axios');
// get all tasks
async function getAllTasks(apiKey, projectId) {
try {
const response = await axios.get(
`https://api.todoist.com/rest/v1/tasks?project_id=${projectId}`,
{
headers: {
Authorization: `Bearer ${apiKey}`,
},
}
);
return response.data;
} catch (error) {
console.error(error);
return null;
}
}
// usage
getAllTasks('YOUR_API_KEY_HERE', 'PROJECT_ID_HERE');
The "getAllTasks" function sends a GET request to the "/tasks" endpoint with the project ID as a query parameter. The API key is included in the request headers. If the request is successful, the function returns the response data, which includes an array of tasks.
Example 3: Update a Task
To update a task in Todoist, you need to send a POST request to the "/tasks/{task_id}" endpoint. Here's how:
const axios = require('axios');
// update a task
async function updateTask(apiKey, taskId, taskData) {
try {
const response = await axios.post(
`https://api.todoist.com/rest/v1/tasks/${taskId}`,
taskData,
{
headers: {
Authorization: `Bearer ${apiKey}`,
},
}
);
return response.data;
} catch (error) {
console.error(error);
return null;
}
}
// usage
updateTask('YOUR_API_KEY_HERE', 'TASK_ID_HERE', {
content: 'Updated Task',
due: {
date: '2022-01-01',
},
});
The "updateTask" function sends a POST request to the "/tasks/{task_id}" endpoint with the updated task data in the request body. The API key is included in the request headers. If the request is successful, the function returns the response data, which includes the updated task information.
Conclusion
In this article, we went through some of the examples of how to use the Todoist API in JavaScript. We showed how to create a new project, get all tasks in a project, and update a task. These examples are just the tip of the iceberg, and you can achieve much more with the Todoist API.
If you want to learn more about the Todoist API, we recommend checking out the API documentation on the Todoist developer website. With this knowledge, you can create robust applications that incorporate the task management features of Todoist.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes