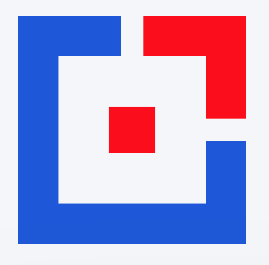
Tomba API
BusinessTomba.io is a website that offers a powerful API for developers to access a wide range of data. With Tomba's API, developers can easily integrate features and functionality into their applications. Tomba provides a simple-to-use interface and extensive documentation to help developers get started quickly. The website offers data on various categories including user data, geo-location data, and more. Tomba's API is designed to be scalable and reliable, making it an ideal choice for businesses of all sizes. Whether you're looking to build a new application or enhance an existing one, Tomba's API provides the flexibility and functionality you need.
📚 Documentation & Examples
Everything you need to integrate with Tomba API
🚀 Quick Start Examples
// Tomba API API Example
const response = await fetch('https://tomba.io/api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Introducing the Tomba Public API
Tomba Public API is a versatile and easy-to-use API endpoint that can be integrated into any web or mobile application. The API provides a wide range of features and functionalities that can be leveraged by developers to enhance the performance and capabilities of their applications.
In this blog post, we will explore the different endpoints of the Tomba Public API and provide various JavaScript code examples to help you get started with this API.
Authentication
Before you can start using the Tomba Public API, you need to authenticate your requests. The API requires an API key to authenticate your requests. You can obtain your API key after you sign up for a Tomba account.
Once you have obtained your API key, you can pass it in the headers of your HTTP requests as follows:
const axios = require('axios');
const API_KEY = 'your-api-key';
const options = {
headers: {
'X-Api-Key': API_KEY
}
};
axios.get('https://api.tomba.io/v1/endpoints', options)
.then(response => console.log(response))
.catch(error => console.log(error));
Endpoints
Tomba Public API covers a wide range of endpoints that can be used to perform various operations, including email verification, lead scoring, and lead enrichment. Here are some examples of the different endpoints and how to use them.
Email Verification
One of the main features of the Tomba Public API is email verification. You can use the email verification endpoint to verify the validity of an email address. Here is an example of how to use the email verification endpoint:
axios.get('https://api.tomba.io/v1/emailVerification?email=test@example.com', options)
.then(response => console.log(response))
.catch(error => console.log(error));
Lead Scoring
Another feature of the Tomba Public API is lead scoring. You can use the lead scoring endpoint to score the lead quality of an email address. Here is an example of how to use the lead scoring endpoint:
axios.get('https://api.tomba.io/v1/leadScoring?email=test@example.com', options)
.then(response => console.log(response))
.catch(error => console.log(error));
Lead Enrichment
The Tomba Public API also provides lead enrichment services. You can use the lead enrichment endpoint to enrich leads with additional data such as company details and social media profiles. Here is an example of how to use the lead enrichment endpoint:
axios.get('https://api.tomba.io/v1/leadEnrichment?email=test@example.com', options)
.then(response => console.log(response))
.catch(error => console.log(error));
Conclusion
In this blog post, we have explored the different endpoints of the Tomba Public API and provided various JavaScript code examples to help you get started with this API. The Tomba Public API is an incredibly powerful tool that can be leveraged to enhance the performance and capabilities of any web or mobile application. If you haven't already, sign up for your Tomba account and start exploring the different endpoints of this incredibly versatile API!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes