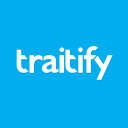
Traitify
PersonalityAssess, collect, and analyze Personality. We understand that behind every user is a human being with a personality waiting to be discovered. With that in mind, we've created a diverse set of fun visual assessments used to uncover personality types and traits. Your users simply select "Me" or "Not Me" to a brief series of images and voila! Instant, actionable data. Our Trait Widgets are a simple and beautiful way to display individual personality traits. Each trait features a unique title and brief description, with the corresponding personality type presented subtly in the background. The colored top bar serves a double purpose of providing additional context with the associated personality type, as well as to visually represent the strength of that specific trait within a user.
π Documentation & Examples
Everything you need to integrate with Traitify
π Quick Start Examples
// Traitify API Example
const response = await fetch('https://app.traitify.com/developer', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using the Traitify API with JavaScript
The Traitify API provides access to a variety of personality and career assessment data. As a developer, you can integrate this data into your applications to provide a more personalized experience to your users.
In this blog post, we'll explore how to use the Traitify API with JavaScript. We'll provide examples of each API endpoint and show you how to retrieve data using AJAX requests.
Getting Started
Before we jump into the code examples, you'll need to sign up for an API key on the Traitify Developer Portal. Once you've created an account, you can create a new project and generate an API key.
Accessing the API
To access the Traitify API, you'll need to include your API key in the HTTP headers of your AJAX requests. Here's an example of how to do that:
const headers = new Headers();
headers.append("Authorization", `Basic ${btoa("secret_key:")}`);
headers.append("Content-Type", "application/json");
const options = {
method: "GET",
headers: headers,
};
fetch("https://api.traitify.com/v1/assessments", options)
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
In this code example, we're using the fetch
function to make a GET request to the /assessments
endpoint of the Traitify API. We've also added the necessary headers to authenticate our request.
Retrieving Assessments
To retrieve existing assessments, you can make a GET request to the /assessments
endpoint. Here's an example of how to do that:
const headers = new Headers();
headers.append("Authorization", `Basic ${btoa("secret_key:")}`);
headers.append("Content-Type", "application/json");
const options = {
method: "GET",
headers: headers,
};
fetch("https://api.traitify.com/v1/assessments", options)
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
This code example will log an array of assessments that have been created in your Traitify account.
Retrieving Career Matches
To retrieve career matches based on an assessment, you can make a GET request to the /careers
endpoint. Here's an example of how to do that:
const headers = new Headers();
headers.append("Authorization", `Basic ${btoa("secret_key:")}`);
headers.append("Content-Type", "application/json");
const options = {
method: "GET",
headers: headers,
};
fetch("https://api.traitify.com/v1/assessments/{assessment_id}/careers", options)
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error(error));
In this code example, replace {assessment_id}
with the ID of the assessment you want to retrieve career matches for. The resulting data object will contain an array of career matches.
Conclusion
By integrating the Traitify API into your JavaScript applications, you can provide personalized assessments and career recommendations to your users. We hope this blog post has helped you get started with using the Traitify API with JavaScript.
If you have any questions or comments, feel free to leave them below!
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes