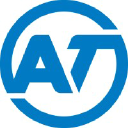
Transport for Auckland, New Zealand
TransportationThe Auckland Transport API is a powerful tool designed to provide developers access to a comprehensive suite of transportation data related to Auckland, New Zealand. This API allows users to integrate real-time public transport information, including bus and train schedules, service disruptions, routes, and other vital transit details into their applications or websites. Through its user-friendly interface, developers can leverage the API's features to enhance user experience by providing accurate and up-to-date transport information, making it an essential resource for any project focused on urban mobility in Auckland. For detailed documentation and implementation guidelines, visit the official documentation at https://dev-portal.at.govt.nz/.
Using the Auckland Transport API offers developers numerous benefits, including seamless integration with existing applications, improved accuracy in transport data, and the ability to deliver real-time updates to end-users. Additionally, the API is designed to be lightweight and efficient, ensuring excellent performance across diverse platforms. Here are some key advantages of using the Auckland Transport API:
- Access to real-time transit data and schedules
- Enhanced user experience through accurate travel information
- Simple integration with various programming languages and frameworks
- Regular updates and maintenance from Auckland Transport for reliability
- Comprehensive documentation and support available for quick implementation
Here's a JavaScript code example for calling the Auckland Transport API:
const fetch = require('node-fetch');
const apiUrl = 'https://api.at.govt.nz/v2/'; // Update with the specific endpoint
const accessToken = 'YOUR_ACCESS_TOKEN'; // Replace with your actual access token
async function getTransportData() {
try {
const response = await fetch(apiUrl, {
method: 'GET',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching transport data:', error);
}
}
getTransportData();