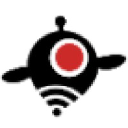
Transport for Belgium
TransportationThe iRail API is a third-party API for Belgian public transport by train
📚 Documentation & Examples
Everything you need to integrate with Transport for Belgium
🚀 Quick Start Examples
// Transport for Belgium API Example
const response = await fetch('https://docs.irail.be/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
The iRail API offers seamless access to information regarding the Belgian public transport train system, making it an essential resource for developers and companies focused on enhancing travel applications. This robust, third-party API provides real-time data about train schedules, statuses, and route information, enabling users to integrate accurate and timely transport insights into their platforms. With a well-documented interface available at iRail Documentation, developers can quickly learn how to harness the power of this API for their applications, ensuring their users have reliable travel information at their fingertips.
By leveraging the iRail API, users can experience numerous benefits. Firstly, developers gain access to real-time train information, enhancing user experience with up-to-date schedules and delays. Secondly, the comprehensive documentation facilitates quick integration, reducing development time. Thirdly, the API supports multiple queries, allowing users to tailor information retrieval according to specific needs. Fourthly, the iRail API promotes efficiency in travel planning, offering direct access to route information and connections. Lastly, it fosters the creation of innovative transport solutions, empowering developers to build smart applications that cater to the evolving needs of travelers.
- Access real-time train schedules and statuses.
- Easily integrate with comprehensive documentation.
- Support for multiple queries tailored to specific needs.
- Enhance travel planning with direct route information.
- Create innovative transport solutions for modern travelers.
Here is a JavaScript code example for calling the iRail API:
fetch('https://api.irail.be/trainboard.php?id=<station_id>&format=json')
.then(response => response.json())
.then(data => {
console.log('Train Information:', data);
})
.catch(error => {
console.error('Error fetching train information:', error);
});
This snippet demonstrates how to fetch train information for a specified station using the iRail API, providing developers with a straightforward method to incorporate Belgian train data into their applications.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes