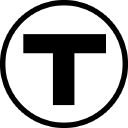
Transport for Boston, US
TransportationMBTA API. The new V3 API provides fast, easy access to MBTA schedules, alerts, and real-time information. The V3 API uses the JSON API format, so you can get started quickly using any of the available libraries V3 API documentation is available using Swagger You can try it out without a key, but we strongly recommend using a key early in the development process. Keys are available for free at api-v3.mbta.com. The V3 API data model is based on GTFS and GTFS-realtime where applicable. The following calls are available: alerts facilities - elevators, escalators, and (coming soon) parking lots, bike racks, etc. predictions - predicted arrival/departure times routes schedules - scheduled arrival/departure times (stop_time) shapes - stops and maps for branches, including route variations stops trips vehicles - vehicle positions
📚 Documentation & Examples
Everything you need to integrate with Transport for Boston, US
🚀 Quick Start Examples
// Transport for Boston, US API Example
const response = await fetch('https://mbta.com/developers/v3-api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Introduction to the MBTA Developers V3 API
The MBTA Developers V3 API is a public API provided by the Massachusetts Bay Transportation Authority (MBTA) that serves real-time data for public transportation modes. The API provides information on elevators, alerts, schedules, and predictions for all transit modes in the greater Boston area.
In this blog post, we will provide several examples of how to use this API in JavaScript.
Getting Started
To begin, we need to retrieve an API key from the MBTA Developer Portal. This key will be used to authenticate our requests to the API. Once we have our key, we can start making requests to the API.
A common use case for the MBTA Developers V3 API is to retrieve real-time train predictions. Here's a code snippet that fetches real-time data for the North Station subway stop:
const API_KEY = 'YOUR_API_KEY_HERE';
const STOP_ID = 'North Station';
fetch(`https://api-v3.mbta.com/predictions?filter[stop]=${STOP_ID}&api_key=${API_KEY}`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In this example, we're using the fetch
method to make a GET request to the /predictions
endpoint of the API. We're passing in our API_KEY
and a filter
parameter that specifies the stop we're interested in. Finally, we're logging the response data to the console.
Parsing the Response
The response from the API contains a lot of information, including predictions for all trains arriving at the specified stop. To make sense of this data, we'll need to parse the response and extract the pieces of information that we're interested in.
Here's an example that extracts the predicted arrival time and the train's direction for the first train in the response:
const API_KEY = 'YOUR_API_KEY_HERE';
const STOP_ID = 'North Station';
fetch(`https://api-v3.mbta.com/predictions?filter[stop]=${STOP_ID}&api_key=${API_KEY}`)
.then(response => response.json())
.then(data => {
const firstTrain = data.data[0];
const arrivalTime = firstTrain.attributes.arrival_time;
const direction = firstTrain.attributes.direction_name;
console.log(`The next train arrives at ${arrivalTime} and is headed ${direction}.`);
})
.catch(error => {
console.error(error);
});
In this example, we're accessing the first train in the response by indexing into the data
array. We're then accessing the arrival_time
and direction_name
attributes of the train object.
Conclusion
In this post, we've covered the basics of using the MBTA Developers V3 API to retrieve real-time data for public transportation in the Boston area. We've provided several examples of how to make API requests and parse the response data in JavaScript.
The API documentation covers many more endpoints and query parameters that you can use to tailor your requests to your specific needs. We encourage you to explore these options and see what the MBTA Developers V3 API can do for you!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes