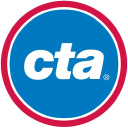
Transport for Chicago, US
TransportationAccessing Public Transit Information with the CTA API
The CTA API provides developers with access to public transit information for the Chicago Transit Authority. This allows developers to create apps that display train and bus schedules, real-time arrival information, and service alerts.
To get started with the CTA API, you'll need an API key. You can register for one at http://www.transitchicago.com/developers/. Once you have your API key, you can start making API requests.
Getting Train and Bus Schedules
To get train schedules, you can use the getTrainSchedule()
function. Here's an example of how to use it in JavaScript:
const api_key = 'your_api_key_here';
const route = 'red'; // specify the train route
const direction = '1'; // specify the direction (1 = south, 5 = north)
const url = `http://lapi.transitchicago.com/api/1.0/ttarrivals.aspx?key=${api_key}&mapid=30000&max=6&rt=${route}&dir=${direction}`;
fetch(url)
.then(response => response.text())
.then(data => console.log(data));
This code will return the next 6 train arrivals for the southbound red line at the 95th/Dan Ryan station.
To get bus schedules, you can use the getBusSchedule()
function. Here's an example of how to use it in JavaScript:
const api_key = 'your_api_key_here';
const route = '20'; // specify the bus route
const stop = '14795'; // specify the bus stop ID
const url = `http://www.ctabustracker.com/bustime/api/v2/getpredictions?key=${api_key}&rt=${route}&stpid=${stop}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
This code will return the next bus arrivals for route 20 at stop 14795.
Accessing Real-Time Train Data
To access real-time train data, you can use the getTrainTracker()
function. Here's an example of how to use it in JavaScript:
const api_key = 'your_api_key_here';
const route = 'red'; // specify the train route
const url = `https://lapi.transitchicago.com/api/1.0/ttpositions.aspx?key=${api_key}&rt=${route}`;
fetch(url)
.then(response => response.text())
.then(data => console.log(data));
This code will return the real-time positions of all trains on the red line.
Accessing Service Alerts
To access service alerts, you can use the getServiceAlerts()
function. Here's an example of how to use it in JavaScript:
const api_key = 'your_api_key_here';
const url = `http://www.transitchicago.com/api/1.0/alerts.aspx?key=${api_key}`;
fetch(url)
.then(response => response.text())
.then(data => console.log(data));
This code will return all service alerts for the CTA.
Conclusion
With the CTA API, developers can access real-time public transit information for the Chicago Transit Authority. By using the code examples demonstrated in this blog post, you can get started building your own public transit apps with ease.