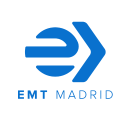
Trasport for Madrid, Spain
TransportationMadrid BUS transport API. EMT Bus Access our bus network: routes, schedules and much more. Move by Bus My itinerary | My bus | My line | Service status | Incidents Access. EMT Mobility Check all the information about the Mobility Aid service. Aid to Mobility. Find your vehicle | Deposit locations | Hours and rates. EMT Parking. Get to know our network of public car parks. Location, hours and rates. Our Parkings My parking | Available places | Rotation | Residents | Sale seats Access BiciMAD. All the information of the BiciMAD service BiciMAD. How it works | Plans & stations | Subscriptions and rates Access Service status . Here you will find incidents and alterations of our service Service status Incidents | Alterations | Other changes Access YOUR BUS AT THE MINUTE Timeouts. Check the expected waiting time for your bus at any stop in the EMT network Calculate your journey. Calculate the optimal bus route between two points in the city of Madrid Lines passing through. Find out which EMT lines pass near where you are.
📚 Documentation & Examples
Everything you need to integrate with Trasport for Madrid, Spain
🚀 Quick Start Examples
// Trasport for Madrid, Spain API Example
const response = await fetch('http://opendata.emtmadrid.es/Servicios-web/BUS', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using the EMT Madrid Public API - Example Code in JavaScript
The EMT Madrid Public API provides real-time information on bus schedules and routes throughout the city of Madrid. In this blog post, we will explore how to access this API with JavaScript, and provide examples of how to retrieve and interpret the provided data.
Requirements
Before we begin, please note that EMT Madrid requires a free API key to access their public API. You can obtain a key by registering on their website at http://opendata.emtmadrid.es/Formulario.
Getting Started
To get started with the EMT Madrid Public API, we need to first obtain data from their API endpoints. Each endpoint corresponds to a specific type of data that can be accessed, such as bus locations or routes.
For example, to retrieve a list of bus routes, you can use the following API endpoint:
http://openapi.emtmadrid.es/v1/transport/busemtmad/routes/
To access this endpoint with JavaScript, we can use the fetch
function, which allows us to send HTTP requests and retrieve the response in our code.
Here's an example code snippet in JavaScript that retrieves the list of bus routes:
fetch('http://openapi.emtmadrid.es/v1/transport/busemtmad/routes/', {
headers: {
'X-API-Key': 'YOUR_API_KEY_HERE'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Note that we need to provide our API key in the X-API-Key
header of our HTTP request, replacing 'YOUR_API_KEY_HERE'
with our actual API key.
Retrieving Bus Locations
Another useful API endpoint provided by EMT Madrid is the ability to retrieve the current locations of active buses in the city.
To access this endpoint, we can use the following URL:
http://openapi.emtmadrid.es/v1/transport/busemtmad/bus/
Again, we need to provide our API key in the X-API-Key
header of our HTTP request. Here's how we can retrieve the list of active bus locations using JavaScript:
fetch('http://openapi.emtmadrid.es/v1/transport/busemtmad/bus/', {
headers: {
'X-API-Key': 'YOUR_API_KEY_HERE'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
In conclusion, the EMT Madrid Public API provides a wealth of information on the bus schedules and routes throughout the city of Madrid. By using our API key and the fetch
function in JavaScript, we can easily retrieve and interpret this data in our applications.
We hope that this blog post has been helpful in demonstrating how to access the EMT Madrid Public API with JavaScript, and provided some useful examples of how to retrieve data from different API endpoints.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes