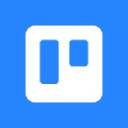
Trello
BusinessThe Trello API offers powerful tools for developers looking to enhance their productivity through seamless project management. With capabilities to create and manipulate boards, lists, and cards, this API is an excellent resource for organizing tasks and prioritizing projects effectively. Utilizing the Trello API can streamline workflows and foster collaboration within teams, allowing for real-time updates and interactions that keep everyone informed and engaged. By integrating Trello's functionality directly into your applications, you can develop robust solutions tailored to your specific project management needs.
Here are five benefits of using the Trello API:
- Effortlessly manage project boards, lists, and cards programmatically.
- Create custom views and workflows suited to your team’s unique requirements.
- Facilitate real-time collaboration through updates and notifications.
- Enhance productivity by automating task management and status tracking.
- Access robust documentation and community support for easier integration.
Here’s a simple JavaScript code example to get you started with the Trello API:
const fetch = require('node-fetch');
const API_KEY = 'your_api_key'; // Replace with your API key
const API_TOKEN = 'your_api_token'; // Replace with your API token
const BOARD_ID = 'your_board_id'; // Replace with the ID of the board you want to access
const url = `https://api.trello.com/1/boards/${BOARD_ID}?key=${API_KEY}&token=${API_TOKEN}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log('Board Title:', data.name);
console.log('Description:', data.desc);
})
.catch(error => {
console.error('Error fetching board:', error);
});