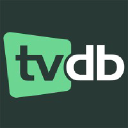
TVDB
VideoThe Television data API provides comprehensive information about a wide variety of TV shows, including episode details, cast and crew information, air dates, and more. This API is an essential resource for developers and businesses looking to enrich their applications with rich television metadata. With its extensive database, it empowers users to easily access and incorporate up-to-date television information into their apps, enhancing user engagement and providing a more informative experience. By utilizing this API, developers can tap into a wealth of content that keeps audiences informed about their favorite shows and upcoming releases.
Benefits of using the Television data API include:
- Access to detailed metadata for thousands of TV series and episodes.
- Regularly updated content to ensure accuracy and relevance.
- Comprehensive search capabilities that allow filtering by genre, network, and air date.
- Easy integration into various applications with a straightforward API structure.
- Support for multiple languages, catering to a global audience.
Here is an example of how to make a call to the Television data API using JavaScript:
const apiKey = 'YOUR_API_KEY'; // Replace with your actual API key
const showId = 'YOUR_SHOW_ID'; // Replace with the ID of the show you want to retrieve
fetch(`https://api.thetvdb.com/series/${showId}`, {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Authorization': apiKey
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching TV data:', error));