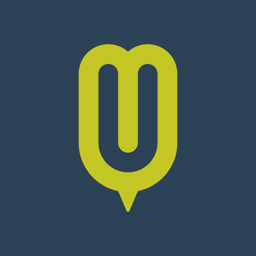
Uebermaps
GeocodingExploring the Uebermaps Public API
Are you looking for an easy way to add maps and location-based functionality to your web or mobile app? Look no further than Uebermaps, a publicly available API that provides access to a wealth of geographic data, including locations, routes, and more.
To get started with the Uebermaps API, you'll need an API key, which you can obtain by signing up for a free account on the Uebermaps website. Once you have your API key, you can start making API calls in your JavaScript code.
Basic API Example
Let's start with a basic example that shows you how to retrieve a list of maps using the Uebermaps API:
const apiKey = "YOUR_API_KEY";
const apiUrl = `https://api.uebermaps.com/v2/maps?api_key=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data));
In this code snippet, we're using the fetch
function to make an HTTP GET request to the Uebermaps API endpoint that retrieves a list of maps. We're passing in our API key as a query parameter in the URL, so that the API knows that we're authorized to access the data. Once the API response is returned, we're converting it to JSON format using the .json()
method, and then logging the resulting data object to the console.
Adding Query Parameters
Most API requests allow you to add additional query parameters to filter and refine the results returned from the API. Here's an example that demonstrates how to query for maps within a certain geographic bounding box:
const apiKey = "YOUR_API_KEY";
const apiUrl = `https://api.uebermaps.com/v2/maps?api_key=${apiKey}&bbox=37.7,-122.5,37.8,-122.4`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data));
In this example, we're adding a bbox
parameter to the API URL, which specifies a geographic bounding box that defines the area we're interested in. The values in the bbox
parameter are latitude and longitude coordinates that define the corners of the bounding box, in the order south,west,north,east
.
Authentication and Authorization
To access Uebermaps API, you'll need to create an account with Uebermaps and generate your own API key. You can then use this key to authenticate all of your API requests and gain access to all the available API functionality.
Conclusion
The Uebermaps API provides developers with an easy and flexible way to add location-based features to their web or mobile apps. With a wide range of query parameters and response formats available, there's no limit to what you can do with this powerful API. Try it out for your next mapping project!