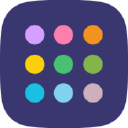
UI Faces
DevelopmentDummy avatar photos and names provided in filterable JSON format. We offer a JSON API which you can use to filter and embed avatars in your application.
π Documentation & Examples
Everything you need to integrate with UI Faces
π Quick Start Examples
// UI Faces API Example
const response = await fetch('https://uifaces.co/api-docs', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Working with the UI Faces API in JavaScript
UI Faces is a free API for generating user avatars. It allows developers to dynamically generate profile images for their products or applications. In this post, we'll take a look at how to use the UI Faces API in JavaScript.
Getting Started
Firstly, you'll need to sign up for an API key on the UI Faces API website. Once you have your API key, you can start making requests to the API endpoints.
Retrieving User Avatars
The most basic endpoint available on the UI Faces API is the GET /api/v2/avatars
endpoint. This endpoint allows you to retrieve a list of user avatars based on certain parameters.
Here's an example of how to fetch a list of user avatars in JavaScript:
const api_key = 'YOUR_API_KEY';
const url = `https://uifaces.co/api/v2/avatars?limit=10`;
fetch(url, {
headers: {
'X-API-KEY': api_key,
},
})
.then((response) => response.json())
.then((data) => console.log(data));
In this example, we're using the fetch
API to make a request to the UI Faces API endpoint. We're passing in our API key as a custom header using the X-API-KEY
header.
The response we get back from this request will be a JSON object containing an array of user avatars.
Advanced Avatar Options
The UI Faces API provides a range of options for customising the avatars that you generate. For example, you can set the gender, age, and ethnicity of the avatar that you want to generate.
Here's an example of how to generate a male avatar with a beard using the GET /api/v2/avatars
endpoint:
const api_key = 'YOUR_API_KEY';
const url = `https://uifaces.co/api/v2/avatars?gender[]=male&limit=1&style[]=beard`;
fetch(url, {
headers: {
'X-API-KEY': api_key,
},
})
.then((response) => response.json())
.then((data) => console.log(data));
In this example, we're passing in the gender
and style
parameters to the endpoint to generate a male avatar with a beard.
Conclusion
The UI Faces API is a simple and powerful way to generate user avatars for your products or applications. By using the JavaScript examples in this post, you'll be able to start integrating the UI Faces API into your own projects.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes