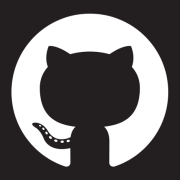
UI Names
DevelopmentUsing the uinames Public API in JavaScript
The uinames API provides a simple way to generate random user names and profile information. It can be useful for testing and development purposes, or for generating data for your own projects. In this article, we'll show you how to use the uinames API in JavaScript.
Getting Started
First, you'll need to make sure that you have a JavaScript environment set up. This could be a web browser, a Node.js server, or another JavaScript environment of your choice.
Next, you can start making requests to the uinames API. The base URL for the API is https://uinames.com/api/
. You can append various endpoints and parameters to this URL to retrieve different types of information.
Retrieving a Single Random User
To retrieve a single random user, you can use the https://uinames.com/api/
endpoint. Here's an example code snippet that demonstrates how to retrieve a random user in JavaScript:
const url = "https://uinames.com/api/";
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
This code uses the fetch()
function to make a request to the uinames API. When a response is received, the response
object is converted to JSON using the .json()
method. Finally, the resulting data is logged to the console.
You should see an output like this:
{
"name": "John",
"surname": "Doe",
"gender": "male",
"region": "United States"
}
This output contains information about a randomly generated user, including the user's name, surname, gender, and region.
Retrieving Multiple Random Users
To retrieve multiple random users, you can use the https://uinames.com/api/?amount=
endpoint. Here's an example code snippet that demonstrates how to retrieve three random users in JavaScript:
const url = "https://uinames.com/api/?amount=3";
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
This code is similar to the previous example, but includes the amount
parameter to retrieve multiple users. The resulting data will contain an array of user objects, like this:
[
{
"name": "John",
"surname": "Doe",
"gender": "male",
"region": "United States"
},
{
"name": "Jane",
"surname": "Doe",
"gender": "female",
"region": "United States"
},
{
"name": "Bob",
"surname": "Smith",
"gender": "male",
"region": "Canada"
}
]
Filtering Results by Country and Gender
You can filter the results of your API requests by specifying a region
and/or gender
parameter. For example, to retrieve three random female users from Brazil, you can use the following code:
const url = "https://uinames.com/api/?amount=3&gender=female®ion=brazil";
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
This will return an array of user objects that match the specified criteria.
Conclusion
The uinames API provides a simple and easy-to-use way to generate random user names and profile information. With a little bit of JavaScript code, you can retrieve and filter this information to meet your needs.