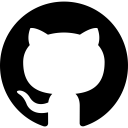
UK Carbon Intensity
EnvironmentThe Official Carbon Intensity API for Great Britain, developed by National Grid, provides real-time data on the carbon intensity of electricity generation across the country. This powerful API is designed to enable developers and organizations to access crucial information about carbon emissions associated with electricity consumption. By leveraging this resource, users can make informed decisions to reduce their carbon footprint, track energy efficiency, and contribute to sustainability efforts. The API features a simple and straightforward interface, allowing easy integration into applications and services, making it an essential tool for anyone interested in energy management and environmental impact.
Utilizing the Carbon Intensity API comes with several benefits, such as enhanced understanding of carbon emissions associated with electricity use, the ability to make data-driven decisions for energy consumption optimization, and real-time updates that provide current carbon intensity levels. Additionally, the API supports a wide range of use cases, including education, research, and operational efficiencies for businesses committed to sustainability. Furthermore, it is freely accessible, empowering developers to innovate and improve environmental awareness among users.
- Access real-time carbon intensity data for Great Britain.
- Make data-driven decisions to optimize energy consumption.
- Monitor the carbon impact of electricity generation.
- Integrate easily into applications and systems.
- Contribute to sustainability initiatives and awareness.
Here is a simple JavaScript code example to call the Carbon Intensity API:
fetch('https://api.carbonintensity.org.uk/intensity')
.then(response => response.json())
.then(data => {
console.log('Current Carbon Intensity Data:', data);
// Further processing of the data can be done here
})
.catch(error => {
console.error('Error fetching data:', error);
});