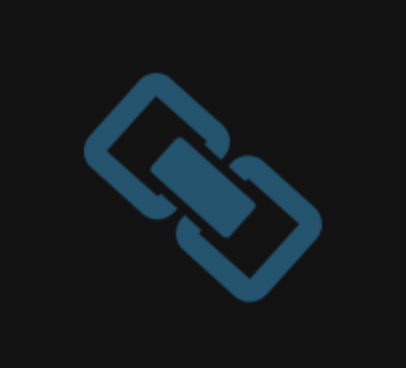
URLmskr
URL ShortenersUsing the UrlMskr API in JavaScript
UrlMskr is a simple and easy-to-use public API that allows you to shorten URLs, unshorten URLs, and retrieve metadata for a given URL. In this blog, we'll go through some example code in JavaScript to demonstrate how to use the UrlMskr API.
Getting Started
Before we can start using the UrlMskr API, we'll need to get an API key. You can get an API key by simply visiting https://urlmskr.com and creating an account.
Once you have an API key, you're ready to start using the API.
Shortening URLs
Shortening a URL is easy with the UrlMskr API. The following code is an example of how to shorten a URL using JavaScript:
fetch('https://api.urlmskr.com/v1/shorten', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'X-ApiKey': 'your_api_key'
},
body: JSON.stringify({url: 'https://example.com'})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're using the fetch
method to send a POST
request to the UrlMskr API's shorten
endpoint. We include our API key in the X-ApiKey
header, and the URL we want to shorten in the request body.
The API will return a JSON object containing the original URL and the shortened URL. In this code, we're simply logging the response to the console, but you can do whatever you like with the response.
Unshortening URLs
Unshortening a URL is just as easy. The following code demonstrates how to unshorten a URL in JavaScript:
fetch('https://api.urlmskr.com/v1/unshorten', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'X-ApiKey': 'your_api_key'
},
body: JSON.stringify({url: 'https://urlmskr.com/abcxyz'})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're sending a POST
request to the UrlMskr API's unshorten
endpoint, including our API key in the X-ApiKey
header, and the shortened URL we want to unshorten in the request body.
Once again, the API will return a JSON object containing the original URL and the unshortened URL.
Retrieving Metadata
Retrieving metadata for a URL can be useful if you want to display information about a link to your users. The following code demonstrates how to retrieve metadata for a URL in JavaScript:
fetch('https://api.urlmskr.com/v1/metadata?url=https://example.com', {
method: 'GET',
headers: {
'X-ApiKey': 'your_api_key'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're sending a GET
request to the UrlMskr API's metadata
endpoint, including our API key in the X-ApiKey
header, and the URL we want to retrieve metadata for as a query parameter.
The API will return a JSON object containing the title, description, and image of the URL.
Conclusion
In this blog, we've covered how to use the UrlMskr API to shorten and unshorten URLs, as well as retrieve metadata for a given URL. By following these examples, you should be able to integrate the UrlMskr API into your JavaScript application without any issues.