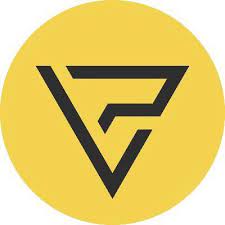
Vertopal
DevelopmentVertopal is a free online tool that allows you to convert a wide range of file types, including pictures, documents, and fonts, to different formats. With Vertopal, you can easily edit and optimize your files, whether you need to resize an image, convert a PDF to a Word document, or extract text from an image. Their user-friendly interface makes it simple to upload and convert files, and you can access the tool from anywhere with an internet connection. Whether you're a student, business owner, or creative professional, Vertopal is an invaluable resource for anyone who needs to manage and manipulate digital files. So why not try it out today and see how easy it can be to convert and edit your files online?
π Documentation & Examples
Everything you need to integrate with Vertopal
π Quick Start Examples
// Vertopal API Example
const response = await fetch('https://www.vertopal.com/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Public API Docs for Vertopal
Vertopal is an API platform that provides a range of data services for businesses. In this article, we'll take a closer look at the Vertopal API and demonstrate how to use it with JavaScript.
Getting Started
First, you'll need to sign up for an API key at https://www.vertopal.com/signup. Once you've done that, you can use your API key to access the various endpoints of the Vertopal API.
API Endpoints
Retrieving Data
To retrieve data from the Vertopal API, you'll make an HTTP GET request to the relevant endpoint. For example, the following code retrieves the latest stock prices for Apple:
const apiKey = 'YOUR_API_KEY_HERE';
const symCode = 'AAPL';
fetch(`https://api.vertopal.com/stocks/latestPrices?symbol=${symCode}&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
Adding Data
To add data to the Vertopal API, you'll make an HTTP POST request to the relevant endpoint. For example, the following code adds a new customer record:
const apiKey = 'YOUR_API_KEY_HERE';
const customerData = {
name: 'John Smith',
email: 'john.smith@example.com',
phone: '+1 (123) 456-7890'
};
fetch('https://api.vertopal.com/customers', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
},
body: JSON.stringify(customerData)
})
.then(response => response.json())
.then(data => console.log(data));
Updating Data
To update data in the Vertopal API, you'll make an HTTP PUT request to the relevant endpoint. For example, the following code updates the email address for an existing customer record:
const apiKey = 'YOUR_API_KEY_HERE';
const customerId = '123456789';
const updatedCustomerData = {
email: 'new.email@example.com'
};
fetch(`https://api.vertopal.com/customers/${customerId}`, {
method: 'PUT',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
},
body: JSON.stringify(updatedCustomerData)
})
.then(response => response.json())
.then(data => console.log(data));
Deleting Data
To delete data from the Vertopal API, you'll make an HTTP DELETE request to the relevant endpoint. For example, the following code deletes a customer record:
const apiKey = 'YOUR_API_KEY_HERE';
const customerId = '123456789';
fetch(`https://api.vertopal.com/customers/${customerId}`, {
method: 'DELETE',
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => console.log(response));
Conclusion
The Vertopal API provides a comprehensive set of data services for businesses, and can be accessed using simple HTTP requests. By using the examples above, you can start using the Vertopal API with JavaScript and integrate it into your projects with ease.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes