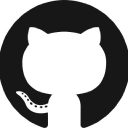
📚 Documentation & Examples
Everything you need to integrate with What's on the menu?
🚀 Quick Start Examples
// What's on the menu? API Example
const response = await fetch('http://nypl.github.io/menus-api/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring the NYPL Menus API
The NYPL Menus API provides access to a large database of historical restaurant menus. With this API, you can search for menus by keyword, browse menus by location, and retrieve detailed information about each menu.
Getting Started
To get started with the NYPL Menus API, you first need to obtain an API key. You can request an API key by visiting the NYPL Digital Collections API website. Once you have your API key, you can use it to make API requests.
Endpoints
The NYPL Menus API has several endpoints for searching and retrieving menus:
Search
The /search
endpoint allows you to search for menus by keyword. For example, to search for menus that contain the word "lobster", you can make the following API request:
const fetch = require('node-fetch');
const apiKey = '<YOUR_API_KEY>';
const query = 'lobster';
const searchUrl = `https://api-ecs.nypl.org/menus/v1/search?q=${query}`;
const options = {
headers: {
'Authorization': `Token token=${apiKey}`,
'Content-Type': 'application/json',
},
};
fetch(searchUrl, options)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This example uses the node-fetch
package to make HTTP requests. The API key is passed in the Authorization
header, and the search query is included in the URL.
Browse
The /browse
endpoint allows you to browse menus by location. For example, to retrieve all menus from New York City, you can make the following API request:
const fetch = require('node-fetch');
const apiKey = '<YOUR_API_KEY>';
const location = 'New York City';
const browseUrl = `https://api-ecs.nypl.org/menus/v1/browse/${location}`;
const options = {
headers: {
'Authorization': `Token token=${apiKey}`,
'Content-Type': 'application/json',
},
};
fetch(browseUrl, options)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This example uses the node-fetch
package to make HTTP requests. The API key is passed in the Authorization
header, and the location is included in the URL.
Get Metadata
The /metadata/:itemId
endpoint allows you to retrieve metadata for a specific menu item. For example, to retrieve metadata for a menu with the ID 9981
, you can make the following API request:
const fetch = require('node-fetch');
const apiKey = '<YOUR_API_KEY>';
const itemId = '9981';
const metadataUrl = `https://api-ecs.nypl.org/menus/v1/metadata/${itemId}`;
const options = {
headers: {
'Authorization': `Token token=${apiKey}`,
'Content-Type': 'application/json',
},
};
fetch(metadataUrl, options)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This example uses the node-fetch
package to make HTTP requests. The API key is passed in the Authorization
header, and the item ID is included in the URL.
Conclusion
The NYPL Menus API provides a rich source of historical restaurant menus that can be explored and analyzed using automated tools. With the sample code provided above, you can start making requests to this API in JavaScript and integrate it into your own applications.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes