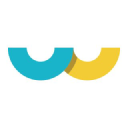
WhereIsMyTransport
TransportationIntroducing WhereIsMyTransport API
WhereIsMyTransport is a public API that provides access to public transport data in various cities across the globe. In this article, we will discuss how to work with this API using JavaScript.
Getting Started
First, you need to create an account on the developer portal of WhereIsMyTransport. Once you have created an account, you can access the API documentation on their website https://developer.whereismytransport.com. The documentation provides detailed information on how to work with the API.
API Endpoints
The WhereIsMyTransport API provides various endpoints for accessing public transport data. Here are a few examples:
1. Finding a Route between two Places
To find a route between two places, you can use the /pt/routers/{router}/plan
endpoint. Here is an example code in JavaScript:
const apiKey = 'your-api-key-here';
const router = 'your-router-code-here';
const fromPlace = '-33.9248685,18.4240553';
const toPlace = '-33.9168655,18.4042062';
fetch(`https://platform.whereismytransport.com/api/schedules?origin=${fromPlace}&destination=${toPlace}&datetime=2021-09-01T08:00:00&api_key=${apiKey}`).then(response => {
return response.json();
}).then(data => {
console.log(data);
}).catch(error => {
console.log(error);
});
In this code, we are using the fetch
API to make a request to the API endpoint. We need to pass the API key and router code in the URL. We also need to pass the origin and destination places in latitude and longitude values. Finally, we are logging the response data in the console.
2. Getting a List of Stops
To get a list of stops in a particular area, you can use the /pt/stops
endpoint. Here is an example code in JavaScript:
const apiKey = 'your-api-key-here';
const latitude = '-33.9248685';
const longitude = '18.4240553';
const radius = '500';
fetch(`https://platform.whereismytransport.com/api/stops?latitude=${latitude}&longitude=${longitude}&radius=${radius}&api_key=${apiKey}`).then(response => {
return response.json();
}).then(data => {
console.log(data);
}).catch(error => {
console.log(error);
});
In this code, we are using the same fetch
API to make a request to the /pt/stops
endpoint. We need to pass the API key and location parameters in the URL. Here, we are passing the latitude and longitude values of the location and a radius of 500 meters to get a list of stops.
Conclusion
In this article, we discussed how to work with the WhereIsMyTransport API using JavaScript. We explored a few examples of API endpoints and how to use them to get public transport data. With the right usage of this API, you can create some useful applications for public transport users.